In the world of Python coding, there are three special helpers: map, filter, and reduce. They’re like handy tools that can make your coding life easier. Map can quickly change a bunch of things, filter can sift through stuff to find what you need, and reduce can combine things into one. Let’s take a closer look at how these simple functions can do some amazing stuff in Python.
Table of Contents
Understanding Map, Filter, and Reduce
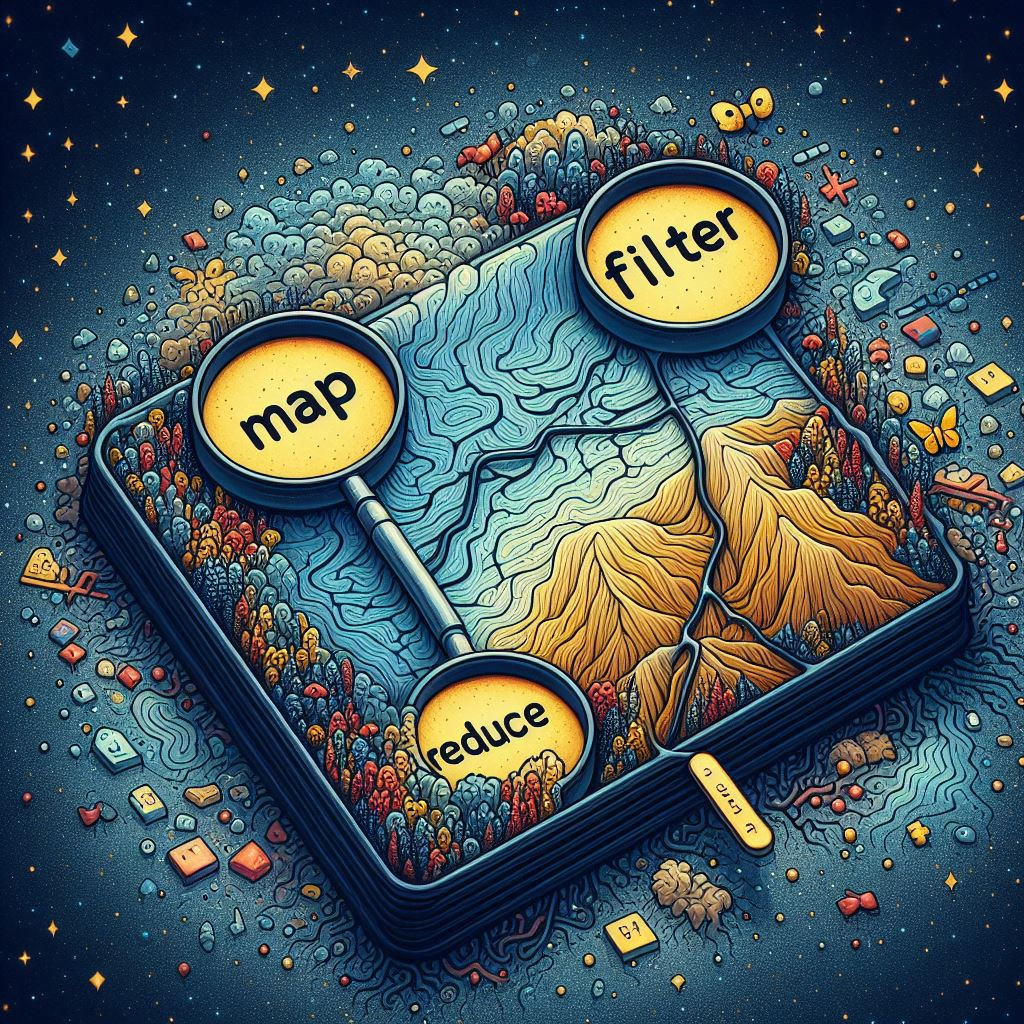
Map
The map function applies a given function to each item in an iterable (such as a list) and returns a new iterable containing the results.
Example 1: Doubling Numbers
Suppose, you have a bunch of numbers and you want to make each number twice as big, you can use the map function to do that.
numbers = [15, 22, 43, 94, 85] doubled_numbers = list(map(lambda x: x * 2, numbers)) print(doubled_numbers) # Output: [30, 44, 86, 188, 170]
Example 2: Converting Strings to Uppercase
Suppose you have a list of names and you want to convert them all to uppercase. You can use the map function with the str.upper method:
names = ["rahul", "john", "aman", "tarun"] uppercase_names = list(map(str.upper, names)) print(uppercase_names) # Output: ['RAHUL', 'JOHN', 'AMAN', 'TARUN']
Example 3: Celsius To Fahrenheit
Consider a scenario where you have a list of temperatures in Celsius and you want to convert them to Fahrenheit. You can use the map function to apply the conversion formula to each temperature.
celsius_temperatures = [0, 10, 20, 30, 40] fahrenheit_temperatures = list(map(lambda c: (c * 9/5) + 32, celsius_temperatures)) print(fahrenheit_temperatures) # Output: [32.0, 50.0, 68.0, 86.0, 104.0]
Filter
The filter function applies a given predicate (a function that returns either True or False) to each item in an iterable and returns a new iterable containing only the items for which the predicate returns True.
Example 1: Filtering Strings by Length
Imagine you’ve got a bunch of words, but you only want to keep the ones that are longer than three letters. You can do this using the filter function and a simple trick called a lambda function.
words = ["rahul", "aman", "henry", "joe", "macie"] long_words = list(filter(lambda x: len(x) > 3, words)) print(long_words) # Output: ['rahul', 'aman', 'henry', 'macie']
Example 2: Filtering Prime Numbers
Imagine you’ve got a bunch of numbers, but you’re only interested in the ones that are prime (that is, only divisible by 1 and themselves). You can use the filter function along with a special trick to check each number and keep only the prime ones.
def is_prime(n): if n <= 1: return False for i in range(2, n): if n % i == 0: return False return True numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] prime_numbers = list(filter(is_prime, numbers)) print(prime_numbers) # Output: [2, 3, 5, 7]
Reduce
The reduce function applies a binary function to the elements of an iterable, progressively combining them into a single value.
Example 1: Summing a List of Numbers
Suppose you have a list of numbers and you want to find their sum using reduce:
from functools import reduce numbers = [1, 2, 3, 4, 5] sum_of_numbers = reduce(lambda x, y: x + y, numbers) print(sum_of_numbers) # Output: 15
Example 2: Finding the Maximum Element in a List
Let’s say you have a list of numbers and you want to find the maximum element using reduce:
from functools import reduce numbers = [3, 7, 2, 9, 5] max_number = reduce(lambda x, y: x if x > y else y, numbers) print(max_number) # Output: 9
Lambda Function: The lambda function (lambda x, y: x if x > y else y)
compares two elements x
and y
. It returns x
if x
is greater than y
, otherwise it returns y
. This lambda function acts as the binary function that is applied to pairs of elements successively.
The reduce
function iterates through the list of numbers pairwise, applying the lambda function to each pair of elements. It starts with the first two elements [3, 7]
, compares them, and returns the greater one (7
). Then it compares this result with the next element (2
), and so on.
As the iteration progresses, the reduce
function progressively combines the elements into a single value based on the logic defined in the lambda function. In this case, it finds the maximum number in the list.
Conclusion
In this blog post, we’ve explored the map, filter, and reduce functions in Python and showcased their real-life applications. Whether you’re manipulating data, performing calculations, or filtering elements, these functions offer efficient and elegant solutions to common programming tasks. By adding map, filter, and reduce to your Python toolkit, you can write cleaner, more expressive code and enhance your coding experience. So, embrace the power of these functions and unlock their potential in your Python projects.