Inheritance is a powerful feature of object-oriented programming (OOP) that allows a class to inherit attributes and methods from another class. Python supports multiple types of inheritance, each serving specific programming needs. In this comprehensive guide, we’ll explore various types of inheritance in Python, accompanied by illustrative examples to deepen your understanding.
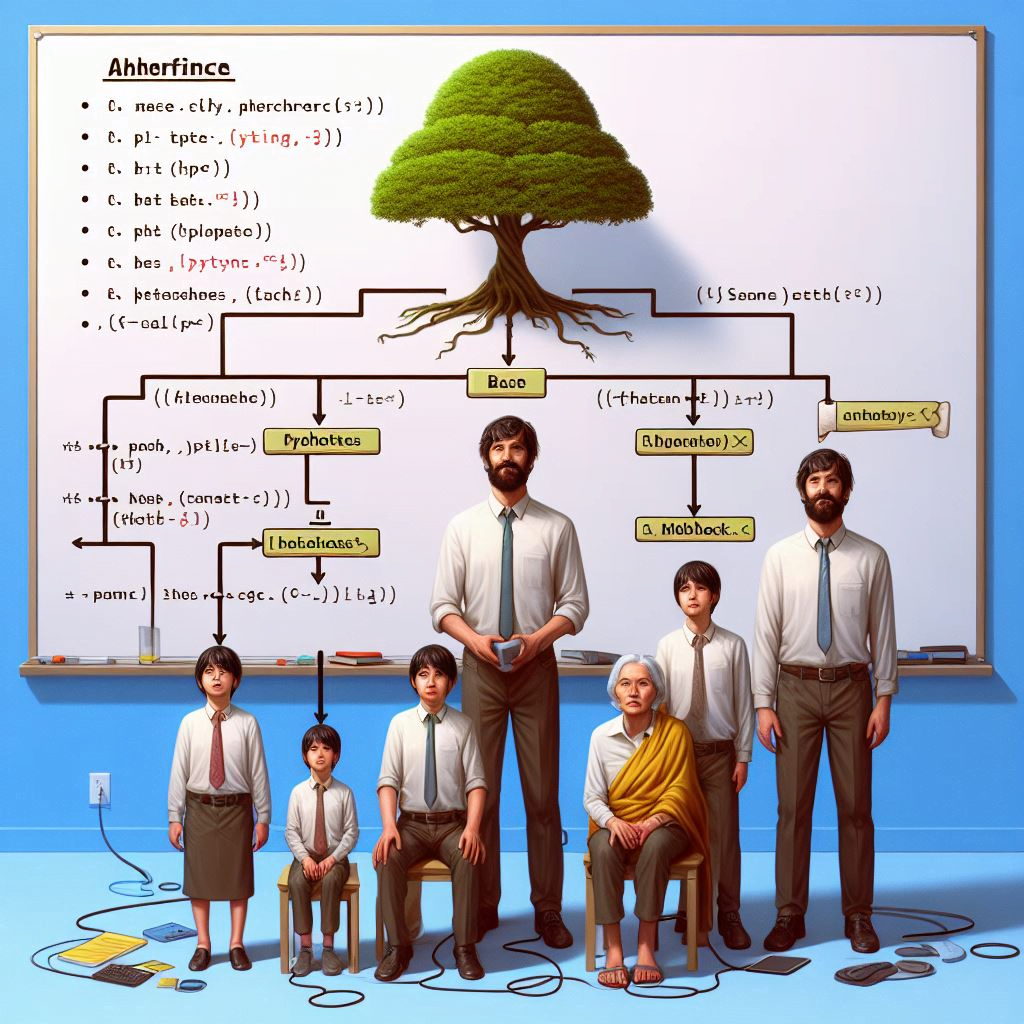
Table of Contents
What is Inheritance?
Inheritance is a fundamental concept in OOP that allows a new class to take on the attributes and behaviors (methods) of an existing class. This facilitates code reuse, enhances maintainability, and promotes the principle of DRY (Don’t Repeat Yourself).
Single Inheritance
Single inheritance means one class inherits from another class. The child class gets all the attributes and methods from its parent class.
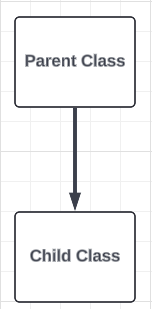
class Parent: def show(self): print("This is parent class") class Child(Parent): def display(self): print("This is child class") # Creating object of child class obj = Child() obj.show() # Output: This is parent class obj.display() # Output: This is child class
Multiple Inheritance
Multiple inheritance happens when a class inherits from two or more parent classes. The child class gets attributes and methods from each of its parent classes.
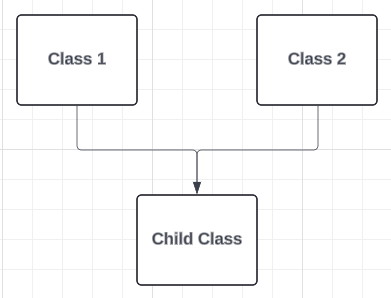
class Class1: def show1(self): print("Method from Class1") class Class2: def show2(self): print("Method from Class2") class Child(Class1, Class2): def display(self): print("Child class method") # Creating object of child class obj = Child() obj.show1() # Output: Method from Class1 obj.show2() # Output: Method from Class2 obj.display() # Output: Child class method
Multilevel Inheritance
In multilevel inheritance, a class inherits from another class, and that class further inherits from another class. It forms a chain of inheritance.
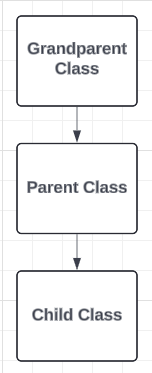
class Grandparent: def show1(self): print("Method from Grandparent") class Parent(Grandparent): def show2(self): print("Method from Parent") class Child(Parent): def display(self): print("Method from Child") # Creating object of child class obj = Child() obj.show1() # Output: Method from Grandparent obj.show2() # Output: Method from Parent obj.display() # Output: Method from Child
Hierarchical Inheritance
Hierarchical inheritance involves one class serving as a parent for multiple child classes.
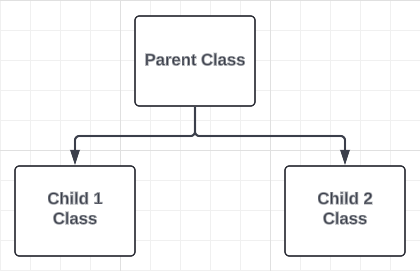
class Parent: def show(self): print("Method from Parent") class Child1(Parent): def display1(self): print("Method from Child1") class Child2(Parent): def display2(self): print("Method from Child2") # Creating objects of child classes obj1 = Child1() obj2 = Child2() obj1.show() # Output: Method from Parent obj1.display1() # Output: Method from Child1 obj2.show() # Output: Method from Parent obj2.display2() # Output: Method from Child2
Hybrid Inheritance
Hybrid inheritance is a combination of any two or more types of inheritance.
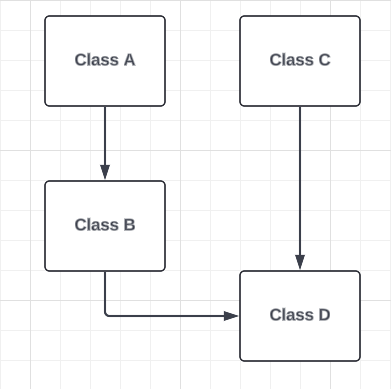
class A: def methodA(self): print("Method from class A") class B(A): def methodB(self): print("Method from class B") class C: def methodC(self): print("Method from class C") class D(B, C): def methodD(self): print("Method from class D") # Creating object of class D obj = D() obj.methodA() # Output: Method from class A obj.methodB() # Output: Method from class B obj.methodC() # Output: Method from class C obj.methodD() # Output: Method from class D
Method Resolution Order (MRO)
In Python, the Method Resolution Order (MRO) defines the sequence in which Python looks for a method in a hierarchy of classes. It can be accessed using the __mro__ attribute.
class A: pass class B(A): pass class C(A): pass class D(B, C): pass print(D.__mro__) # Output: (<class '__main__.D'>, <class '__main__.B'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>)
Conclusion
Inheritance is like passing down traits from parents to children, but in the world of programming. It’s a handy tool in Python that helps developers write code more efficiently.
We’ve learned about different types of inheritance. Single inheritance is when a child class inherits from just one parent class. Multiple inheritance is when a child class gets features from more than one parent class. Multilevel inheritance is like a family tree, where children inherit from their parents, who also inherited from their own parents.
Hierarchical inheritance is when multiple child classes share the same parent class. And Hybrid inheritance is like mixing and matching different types of inheritance.
Understanding these concepts helps developers organize their code better and make it easier to manage and reuse. We’ve also talked about the Method Resolution Order (MRO), which is like a roadmap that Python uses to figure out which part of the code to use when there are overlapping features.
By mastering inheritance, Python developers can create programs that are easier to understand, maintain, and expand. It’s like having a set of building blocks that can be used and reused to build all sorts of amazing things in the world of programming.