Python, known for its simplicity and readability, offers a variety of built-in data structures to facilitate efficient programming. While lists are widely recognized, tuples are another essential data structure in Python that offers unique features and advantages. In this comprehensive guide, we’ll delve into the basics of Python tuples and explore advanced operations to help you master this powerful data structure.
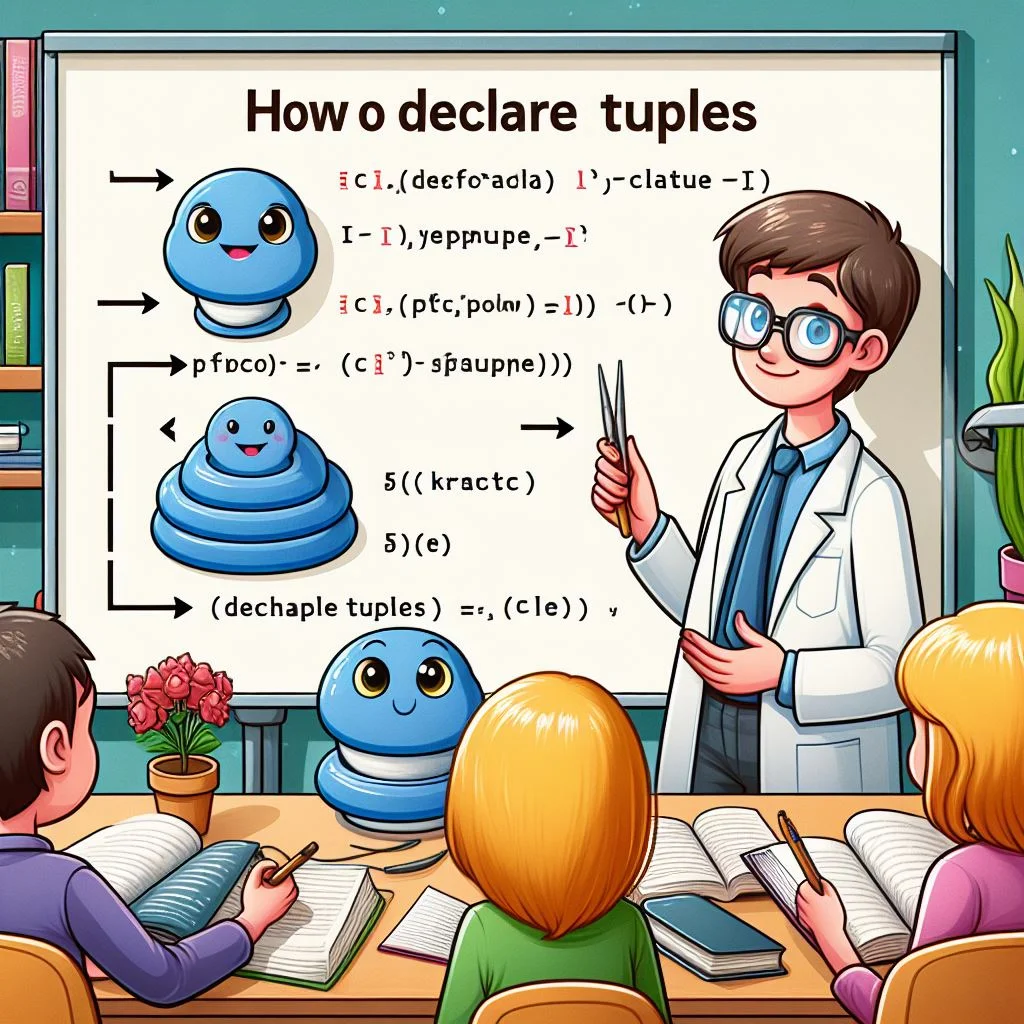
Table of Contents
- What is Python Tuple?
- Basic Operations on Python Tuples
- Advanced Operations on Python Tuples
- Tuple Methods
- Built-in Functions
- Tuple Unpacking
- Tuple Concatenation and Repetition
- Tuple Membership and Iteration
- Tuple Slicing
- Tuple Sorting and Reversing
- Tuple Packing
- Tuple Unpacking with Extended Unpacking
- Tuple to List Conversion and Vice Versa
- Tuple with Single Element
- Tuple Comparison
- Tuple as Dictionary Keys
- Tuple Comprehensions (Generator Expressions)
- Named Tuples
- Tuple Concatenation with ‘+=’
- Count Occurrences with collections.Counter
- Conclusion
What is Python Tuple?
A tuple in Python is an ordered collection of items, similar to a list. However, unlike lists, tuples are immutable, meaning once a tuple is created, you cannot modify its contents. Tuples are typically used to store a collection of related data that should not be changed throughout the program’s execution.
# Example of a Python Tuple my_tuple = (1, 2, 3, 'hello', 'world')
Basic Operations on Python Tuples
Creating a Tuple
Creating a tuple is straightforward. You can initialize an empty tuple or a tuple with elements using parentheses.
# Empty Tuple empty_tuple = () # Tuple with elements numbers = (1, 2, 3, 4, 5)
Accessing Elements
You can access individual elements of a tuple using indexing, similar to python list.
# Accessing elements print(numbers[0]) # Output: 1 print(numbers[2]) # Output: 3
Immutable Nature
Unlike lists, tuples are immutable, meaning you cannot modify the elements of a tuple after it’s created.
# Attempting to modify a tuple (will raise an error) numbers[0] = 10 # TypeError: 'tuple' object does not support item assignment
Advanced Operations on Python Tuples
Tuple Methods
Although tuples are immutable, they have a few methods that can be used for various operations such as counting occurrences of an element or finding the index of an element.
# Tuple methods my_tuple = (1, 2, 3, 4, 2) print(my_tuple.count(2)) # Output: 2 print(my_tuple.index(3)) # Output: 2
Built-in Functions
Python offers several built-in functions that can be used with tuples, such as len(), min(), and max().
# Built-in functions print(len(numbers)) # Output: 5 print(min(numbers)) # Output: 1 print(max(numbers)) # Output: 5
Tuple Unpacking
Tuple unpacking allows you to assign the elements of a tuple to multiple variables in a single statement.
# Tuple unpacking a, b, c = (1, 2, 3) print(a, b, c) # Output: 1 2 3
Tuple Concatenation and Repetition
You can concatenate two tuples using the ‘+’ operator and repeat a tuple using the ‘*’ operator.
# Concatenation and Repetition tuple1 = (11, 22, 33) tuple2 = (44, 55, 66) concatenated_tuple = tuple1 + tuple2 print(concatenated_tuple) # Output: (11, 22, 33, 44, 55, 66) repeated_tuple = tuple1 * 3 print(repeated_tuple) # Output: (11, 22, 33, 11, 22, 33, 11, 22, 33)
Tuple Membership and Iteration
You can check if an element exists in a tuple using the ‘in’ and ‘not in’ operators. Additionally, you can iterate over a tuple using a ‘for’ loop to perform operations on each element.
# Tuple membership print(3 in numbers) # Output: True print(7 not in numbers) # Output: True # Tuple iteration for item in numbers: print(item)
Tuple Slicing
Similar to lists, you can use slicing to access a portion of a tuple by specifying a start index, an end index, and an optional step.
# Tuple slicing letters = ('a', 'b', 'c', 'd', 'e') print(letters[1:4]) # Output: ('b', 'c', 'd')
Tuple Sorting and Reversing
Although tuples are immutable and cannot be sorted or reversed in-place, you can create a new sorted or reversed tuple using the sorted() and reversed() functions.
# Sorting and Reversing numbers = ( 3, 1, 4, 1, 5, 9) sorted_numbers = tuple(sorted(numbers)) print(sorted_numbers) # Output: (1, 1, 3, 4, 5, 9) reversed_numbers = tuple(reversed(numbers)) print(reversed_numbers) # Output: (9, 5, 1, 4, 1, 3)
Tuple Packing
Tuple packing refers to the process of packing multiple values into a single tuple. This happens implicitly when you assign multiple values to a single variable separated by commas.
# Tuple packing packed_tuple = 1, 'apple', 3.14 print(packed_tuple) # Output: (1, 'apple', 3.14)
Tuple Unpacking with Extended Unpacking
You can also use extended unpacking to assign multiple values from a tuple to multiple variables, including using the ‘*’ operator to capture remaining elements.
# Extended tuple unpacking a, *b, c = (1, 2, 3, 4, 5) print(a) # Output: 1 print(b) # Output: [2, 3, 4] print(c) # Output: 5
Tuple to List Conversion and Vice Versa
You can convert a tuple to a list using the list() constructor and convert a list to a tuple using the tuple() constructor.
tuple_to_list = tuple1 print(list(tuple_to_list)) # Output: [11, 22, 33] list_to_tuple = tuple(tuple_to_list) print(list_to_tuple) # Output: (11, 22, 33)
Tuple with Single Element
If you want to create a tuple with a single element, you need to include a trailing comma to distinguish it from a regular parentheses expression.
# Tuple with single element single_element_tuple = (42,) print(single_element_tuple) # Output: (42,)
Tuple Comparison
You can compare tuples using comparison operators (<, <=, >, >=, ==, !=). Tuples are compared element-wise from left to right, and the comparison stops as soon as a mismatch is found.
# Tuple comparison tuple1 = (1, 2, 3) tuple2 = (1, 2, 4) print(tuple1 < tuple2) # Output: True
Tuple as Dictionary Keys
Since tuples are immutable and hashable (if they contain only hashable elements), they can be used as keys in dictionaries.
# Tuple as dictionary keys my_dict = {(1, 2): 'apple', (3, 4): 'banana'} print(my_dict[(1, 2)]) # Output: 'apple'
Tuple Comprehensions (Generator Expressions)
Similar to list comprehensions, you can use generator expressions to create tuples.
# Tuple comprehension (generator expression) numbers = (x for x in range(5)) print(tuple(numbers)) # Output: (0, 1, 2, 3, 4)
Named Tuples
Named tuples are tuple subclasses that have named fields. They can be a more readable alternative to ordinary tuples.
from collections import namedtuple # Define a named tuple Person = namedtuple('Person', ['name', 'age']) # Create an instance person = Person(name='John', age=30) print(person.name) # Output: 'John' print(person.age) # Output: 30
Tuple Concatenation with ‘+=’
You can use the ‘+=’ operator to concatenate tuples, similar to lists.
# Tuple concatenation with += tuple1 = (11, 22, 33) tuple2 = (44, 55, 66) tuple1 += tuple2 print(tuple1) # Output: (11, 22, 33, 44, 55, 66)
Count Occurrences with collections.Counter
You can use the Counter class from the collections module to count occurrences of elements in a tuple.
from collections import Counter # Count occurrences numbers = (1, 2, 3, 2, 1, 3, 4, 5) counter = Counter(numbers) print(counter) # Output: Counter({1: 2, 2: 2, 3: 2, 4: 1, 5: 1})
Conclusion
Python tuples are a versatile and powerful data structure that offers a range of operations suitable for various programming needs. From basic operations like accessing elements and tuple slicing to advanced techniques like tuple unpacking, sorting, and extended unpacking, tuples provide a comprehensive set of tools for efficient data manipulation.
Whether you’re working with data that should remain unchanged throughout your program or leveraging the unique features of tuples for specific tasks, understanding these advanced operations will help you make the most out of Python tuples in your programming journey. With this expanded guide, you’re well-prepared to utilize the full potential of tuples in your Python projects. Happy coding!