In the realm of high-level programming languages like Python, the Python list stands out as a versatile and widely-used built-in data structure. In this comprehensive guide, we’ll delve into the intricacies of Python lists, covering everything from basic operations to advanced techniques, empowering you with a thorough understanding of this fundamental data structure.
Table of Contents
What is a Python List?
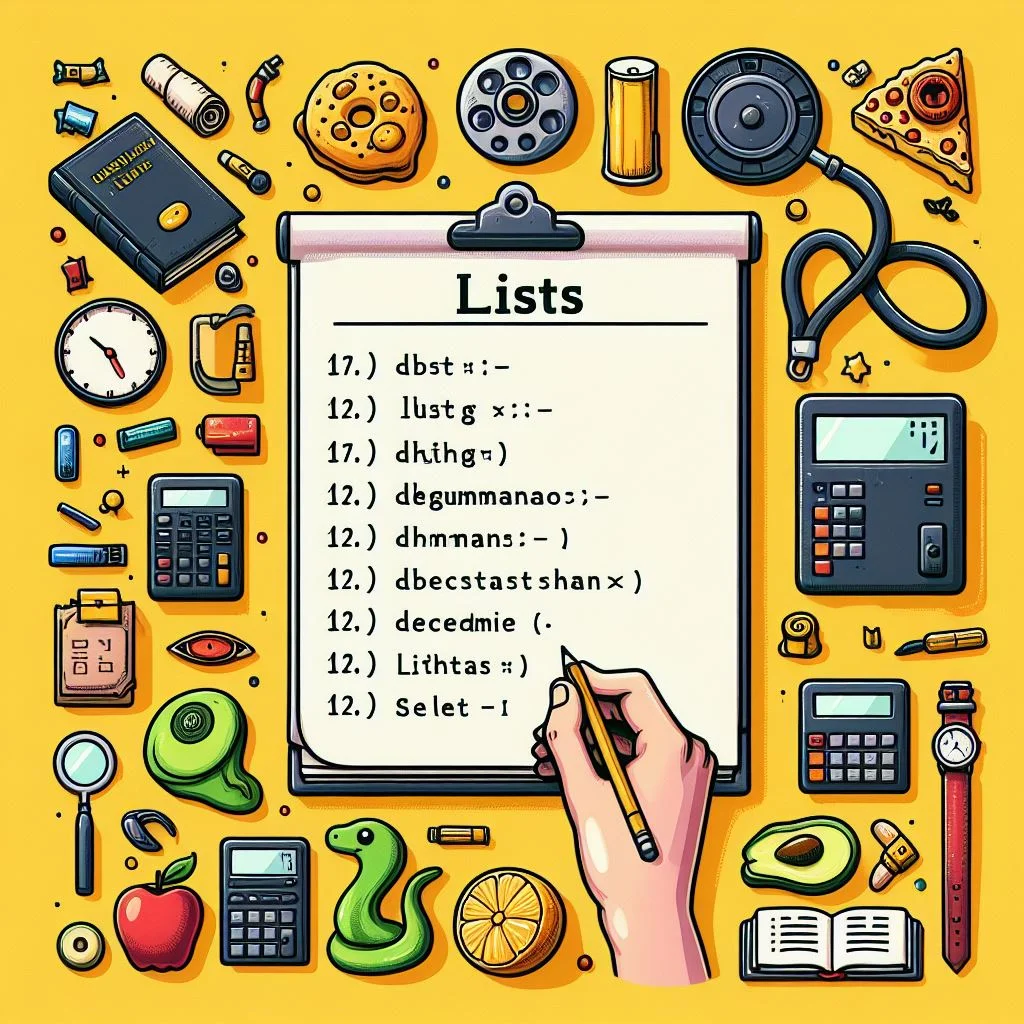
A list in Python is an ordered collection of items, where each item is identified by an index. Lists are versatile, allowing you to store and manipulate heterogeneous data types, including integers, floats, strings, and even other lists.
# Example of a Python List my_list = [10, 23, 45, 67, 'hello', 'world']
Basic Operations on Lists
Creating a List
Creating a list is straightforward. You can initialize an empty list or a list with elements using square brackets.
# Empty List empty_list = [] # List with elements numbers = [10, 23, 45, 67, 89]
Accessing Elements
You can retrieve individual elements from a list using indexing. In Python, indexing starts from zero, indicating that the first element is located at index 0.
# Accessing elements print(numbers[0]) # Output: 10 print(numbers[2]) # Output: 45
Modifying Elements
Lists are mutable, allowing you to change individual elements within them.
# Modifying elements numbers[0] = 100 print(numbers) # Output: [100, 23, 45, 67, 89]
Adding Elements
You can append elements to the end of a list using the append()
method or insert elements at a specific position using the insert()
method.
# Adding elements numbers.append(90) print(numbers) # Output: [100, 23, 45, 67, 89, 90] numbers.insert(1, 15) print(numbers) # Output: [100, 15, 23, 45, 67, 89, 90]
Removing Elements
To remove elements, you can use the remove()
method to delete a specific value or the pop()
method to remove an element at a specified index.
# Removing elements numbers.remove(15) print(numbers) # Output: [100, 23, 45, 67, 89, 90] numbers.pop(0) print(numbers) # Output: [23, 45, 67, 89, 90]
List Slicing
List slicing allows you to access a portion of a list by specifying a start index, an end index, and an optional step.
# List slicing letters = ['a', 'b', 'c', 'd', 'e'] print(letters[1:4]) # Output: ['b', 'c', 'd']
Advanced Operations on Lists
List Comprehensions
List comprehensions are a powerful feature in Python that allows you to create new lists based on existing iterables, such as lists, strings, or range objects. They provide a concise and readable way to generate lists without the need for traditional loops.
#Suppose you have a list of temperatures in Celsius and you want to convert them to Fahrenheit using the formula (Celsius * 9/5) + 32. # Using list comprehension celsius_temps = [0, 10, 20, 30, 40] fahrenheit_temps = [(temp * 9/5) + 32 for temp in celsius_temps] print(fahrenheit_temps) # Output: [32.0, 50.0, 68.0, 86.0, 104.0]
Nested Lists
A list can contain other lists, allowing you to create nested data structures.
# Nested lists matrix = [[11, 22, 33], [44, 55, 66], [77, 88, 99]] print(matrix[1][1]) # Output: 55
List Methods
Python provides various built-in methods for manipulating lists, such as sort()
, reverse()
, and count()
.
# List methods numbers.sort() print(numbers) # Output: [23, 45, 67, 89, 90] numbers.reverse() print(numbers) # Output: [90, 89, 67, 45, 23] print(numbers.count(67)) # Output: 1
Built-In Functions
Python also offers built-in functions like len()
, min()
, and max()
that can be used with lists.
# Built-in functions print(len(numbers)) # Output: 5 print(min(numbers)) # Output: 23 print(max(numbers)) # Output: 90
Sorting and Reversing
You can sort a list using the sorted()
function, which returns a new sorted list without modifying the original list.
# Sorting sorted_numbers = sorted(numbers) print(sorted_numbers) # Output: [23, 45, 67, 89, 90]
List Copying
Be cautious when copying lists as simply assigning one list to another creates a reference, not a copy.
# Copying lists new_numbers = numbers.copy() print(new_numbers) # Output: [90, 89, 67, 45, 23]
List Concatenation and Repetition
You can concatenate two lists using the +
operator and repeat a list using the *
operator.
# Concatenation and Repetition list1 = [10, 20, 30] list2 = [40, 50, 60] concatenated_list = list1 + list2 print(concatenated_list) # Output: [10, 20, 30, 40, 50, 60] repeated_list = list1 * 3 print(repeated_list) # Output: [10, 20, 30, 10, 20, 30, 10, 20, 30]
List Membership
You can check if an element exists in a list using the in
and not in
operators.
# List membership print(45 in numbers) # Output: True print(100 not in numbers) # Output: True
List Iteration
You can iterate over a list using a for
loop to perform operations on each element.
# List iteration for num in numbers: print(num)
Conclusion
Python lists are versatile and powerful data structures that can handle a wide range of tasks. Whether you’re a beginner or an experienced Python developer, understanding the basic and advanced operations on python list is essential for efficient and effective programming.
In this comprehensive guide, we covered the basics of Python lists, including creating lists, accessing elements, modifying elements, adding and removing elements, and list slicing. These fundamental operations provide a solid foundation for working with lists in Python.
We then delved into more advanced techniques such as list comprehensions, which offer a concise and readable way to create new lists based on existing iterables. With list comprehensions, you can perform complex operations in a single line of code, making your programs more efficient and easier to understand.
We also explored other advanced operations on lists, including nested lists, list methods, built-in functions, sorting, reversing, copying, concatenation, repetition, membership, and iteration. These operations allow you to manipulate lists in various ways to suit your specific requirements.
In conclusion, Python lists are a fundamental part of the language and provide a flexible and efficient way to store and manipulate data. By mastering the operations and techniques covered in this guide, you’ll be well-equipped to leverage the full potential of Python lists in your projects, whether you’re building simple scripts or complex applications. Happy coding!