Python dictionaries, one of the most dynamic and widely-used data structures in Python, offer a versatile and powerful tool for efficient and flexible programming. In this comprehensive guide, we’ll delve into the basics of Python dictionaries and explore advanced operations to help you harness the full potential of this indispensable data structure.
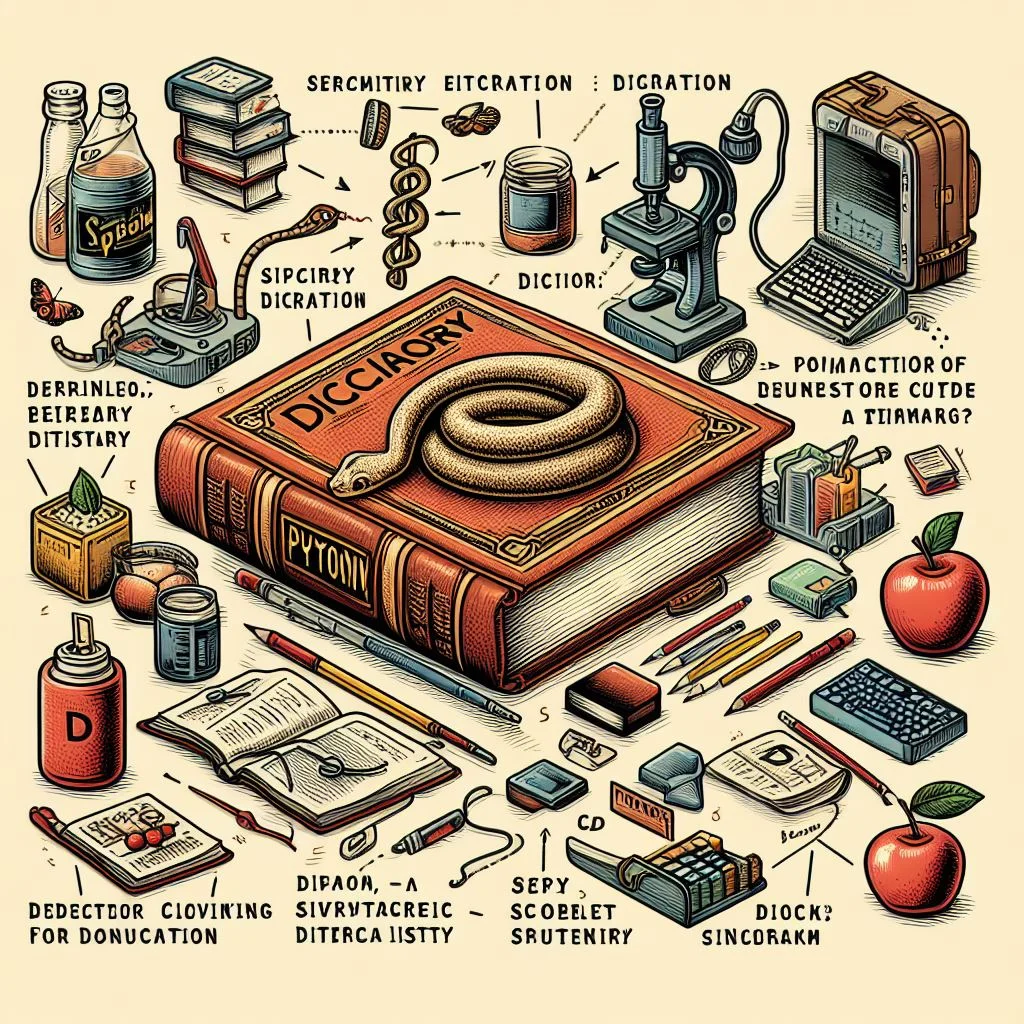
Table of Contents
- What is Python Dictionary?
- Basic Operations on Python Dictionaries
- Advanced Operations on Dictionaries
- Dictionary Methods
- Built-in Functions
- Dictionary Comprehensions
- Dictionary Iteration
- Dictionary Views
- Nested Dictionaries
- Merging Dictionaries
- Dictionary Sorting
- Dictionary Membership
- Dictionary Copying
- Dictionary Aliasing
- Dictionary get() Method
- Dictionary setdefault() Method
- Dictionary clear() Method
- Dictionary fromkeys() Method
- Dictionary popitem() Method
- Dictionary update() Method with Iterable of Key-Value Pairs
- Conclusion
What is Python Dictionary?
A dictionary in Python is a collection of key-value pairs where keys are unique and mapped to corresponding values. Each key is unique, and it maps to a corresponding value. Dictionaries are mutable, meaning you can modify them after creation, making them incredibly versatile for a wide range of applications.
# Example of a Python Dictionary my_dict = {'name': 'Tarun', 'age': 23, 'city': 'Ghaziabad'}
Basic Operations on Python Dictionaries
Creating a Dictionary
Creating a dictionary is straightforward. You can initialize an empty dictionary or a dictionary with key-value pairs using curly braces {}.
# Empty Dictionary empty_dict = {} # Dictionary with elements my_dict = {'name': 'Tarun', 'age': 23, 'city': 'Ghaziabad'}
Accessing Elements
You can access the value associated with a key by using the key within square brackets.
# Accessing elements print(my_dict['name']) # Output: 'Tarun' print(my_dict['age']) # Output: 23
Modifying Elements
Dictionaries are mutable, so you can update the value associated with a key.
# Modifying elements my_dict['age'] = 24 print(my_dict) # Output: {'name': 'Tarun', 'age': 24, 'city': 'Ghaziabad'}
Adding Elements
You can append new key-value pairs to a dictionary.
# Adding elements my_dict['job'] = 'Engineer' print(my_dict) # Output: {'name': 'Tarun', 'age': 24, 'city': 'Ghaziabad', 'job': 'Engineer'}
Removing Elements
You can remove a key-value pair from a dictionary using the del keyword or the pop() method.
# Removing elements del my_dict['job'] print(my_dict) # Output: {'name': 'Tarun', 'age': 24, 'city': 'Ghaziabad'} my_dict.pop('age') print(my_dict) # Output: {'name': 'Tarun', 'city': 'Ghaziabad'}
Dictionary Length
You can get the number of key-value pairs in a dictionary using the len() function.
# Dictionary length print(len(my_dict)) # Output: 2
Advanced Operations on Dictionaries
Dictionary Methods
Python provides various built-in methods for manipulating dictionaries, such as keys(), values(), and items().
# Dictionary methods print(my_dict.keys()) # Output: dict_keys(['name', 'city']) print(my_dict.values()) # Output: dict_values(['Tarun', 'Ghaziabad']) print(my_dict.items()) # Output: dict_items([('name', 'Tarun'), ('city', 'Ghaziabad')])
Built-in Functions
Python offers built-in functions like len(), sorted(), and sum() that can be used with dictionaries.
# Built-in functions print(len(my_dict)) # Output: 2 print(sorted(my_dict.keys()))# Output: ['city', 'name']
Dictionary Comprehensions
Similar to list comprehensions, you can use dictionary comprehensions to create dictionaries in a concise manner.
# Dictionary comprehension squares = {x: x*x for x in (2, 3, 4)} print(squares) # Output: {2: 4, 3: 9, 4: 16}
Dictionary Iteration
You can iterate over a dictionary using a for loop to access keys, values, or key-value pairs.
# Dictionary iteration for key in my_dict: print(key, my_dict[key])
Dictionary Views
Dictionary views (keys(), values(), items()) provide dynamic views on the dictionary’s contents, allowing you to observe changes.
# Dictionary views keys_view = my_dict.keys() my_dict['job'] = 'Engineer' print(keys_view) # Output: dict_keys(['name', 'city', 'job'])
Nested Dictionaries
Dictionaries can contain other dictionaries, allowing you to create nested data structures.
# Nested dictionaries employees = { 'employee1': {'name': 'John', 'age': 30}, 'employee2': {'name': 'Jane', 'age': 25} }
Merging Dictionaries
You can merge two dictionaries using the update() method or dictionary unpacking.
# Merging dictionaries dict1 = {'a': 11, 'b': 22} dict2 = {'b': 33, 'c': 44} dict1.update(dict2) print(dict1) # Output: {'a': 11, 'b': 33, 'c': 44}
Dictionary Sorting
While dictionaries are inherently unordered, you can sort them by keys or values using the sorted() function.
# Dictionary sorting sorted_dict = dict(sorted(my_dict.items(), key=lambda x: x[0])) print(sorted_dict) # Output: {'city': 'Ghaziabad', 'job': 'Engineer', 'name': 'Tarun'}
Dictionary Membership
You can check if a key exists in a dictionary using the in and not in operators.
# Dictionary membership print('name' in my_dict) # Output: True print('job' not in my_dict) # Output: True
Dictionary Copying
Be cautious when copying dictionaries as simply assigning one dictionary to another creates a reference, not a copy.
# Copying dictionaries new_dict = my_dict.copy() print(new_dict) # Output : {'name': 'Tarun', 'city': 'Ghaziabad', 'job': 'Engineer'}
Dictionary Aliasing
When you assign a dictionary to another variable, both variables refer to the same memory location. Any changes made to one variable will reflect in the other.
# Dictionary aliasing alias_dict = my_dict alias_dict['city'] = 'Los Angeles' print(my_dict) # Output: {'name': 'Tarun', 'city': 'Los Angeles', 'job': 'Engineer'}
Dictionary get() Method
The get() method allows you to retrieve the value for a specified key. If the key does not exist, it returns a default value, which is None by default.
# Using get() method print(my_dict.get('name')) # Output: 'Tarun' print(my_dict.get('salary')) # Output: None print(my_dict.get('salary', 0))# Output: 0
Dictionary setdefault() Method
The setdefault() method retrieves the value of a key from the dictionary. If the key is not present, it adds the key with a designated value.
# Using setdefault() method print(my_dict.setdefault('name', 'Jane')) # Output: 'Jane' print(my_dict.setdefault('salary', 5000)) # Output: 5000 print(my_dict) # Output: {'name': 'Tarun', 'city': 'Los Angeles', 'job': 'Engineer', 'salary': 5000}
Dictionary clear() Method
The clear() method removes all items from the dictionary.
# Using clear() method my_dict.clear() print(my_dict) # Output: {}
Dictionary fromkeys() Method
The fromkeys() method creates a new dictionary with keys from a sequence and values set to a specified value.
# Using fromkeys() method keys = ['name', 'age', 'city'] default_value = 'Unknown' new_dict = dict.fromkeys(keys, default_value) print(new_dict) # Output: {'name': 'Unknown', 'age': 'Unknown', 'city': 'Unknown'}
Dictionary popitem() Method
The popitem() method removes and returns the last/latest key-value pair added to the dictionary.
# Using popitem() method person = {'name': 'Tarun', 'age': 23, 'city': 'Ghaziabad'} print(person.popitem()) # Output: ('city', 'Ghaziabad') print(person) # Output: {'name': 'Tarun', 'age': 23}
Dictionary update() Method with Iterable of Key-Value Pairs
The update() method can also accept an iterable of key-value pairs.
# Using update() method with an iterable of key-value pairs person.update([('city', 'Los Angeles'), ('job', 'Engineer')]) print(person) # Output: {'name': 'Tarun', 'age': 23, 'city': 'Los Angeles', 'job': 'Engineer'}
Conclusion
Python dictionaries are incredibly versatile and offer a wide array of operations and methods to manipulate key-value pairs efficiently. From the basics of creating, accessing, and modifying dictionaries to advanced techniques like aliasing, using get(), setdefault(), and more, dictionaries provide a robust set of tools for various programming needs.
Understanding these advanced operations will further enhance your proficiency in using dictionaries effectively in Python. With this comprehensive guide, you’re well-equipped to leverage the full capabilities of dictionaries in your Python projects. Happy coding!