In Django, a proxy model is a way to create a different Python class with the same database table and fields as an existing model. Proxy models are useful when you want to change the behavior of a model without changing the original model’s fields or methods. Here’s a complete example to demonstrate how to create and use a proxy model in Django.
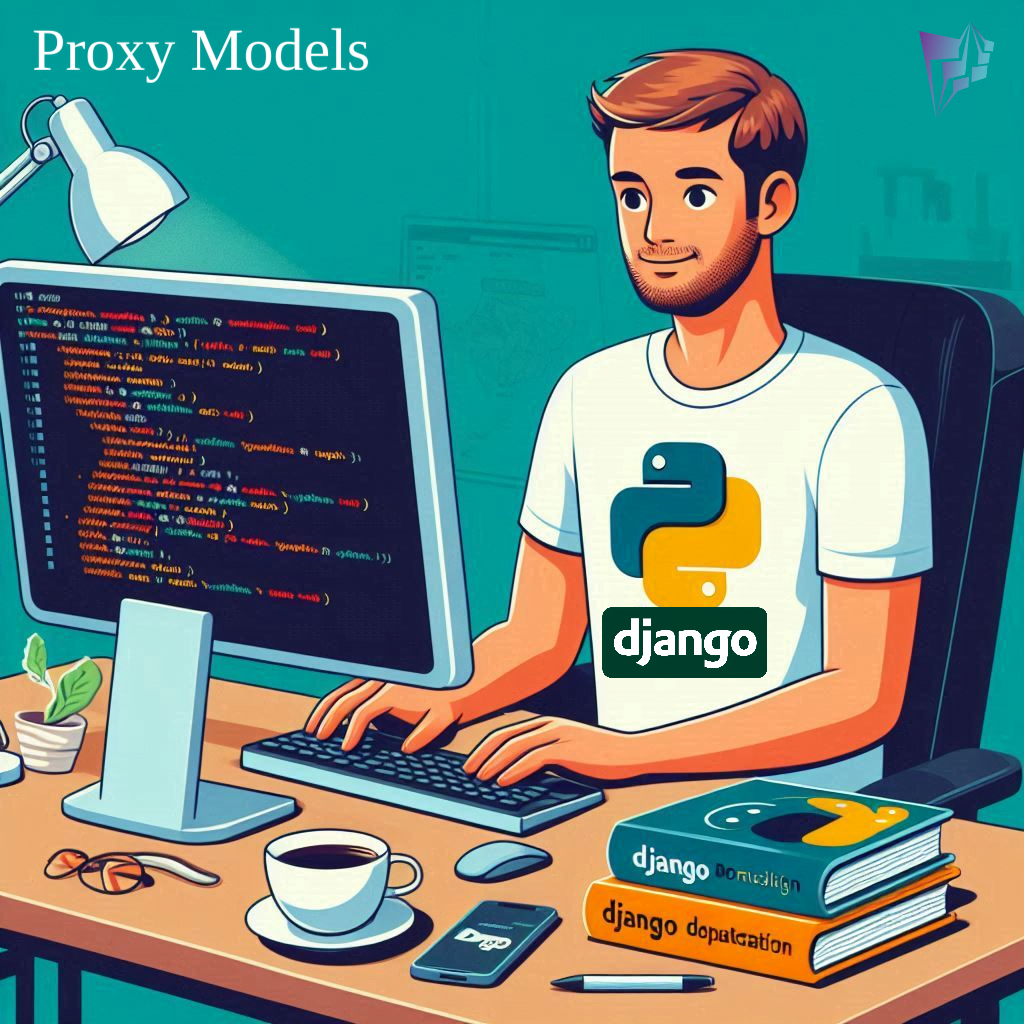
Suppose we have a simple Django app named myapp
with an existing model called Person
:
- Original Model (
Person
): This is the model we want to proxy.
# app/models.py from django.db import models class Person(models.Model): first_name = models.CharField(max_length=50) last_name = models.CharField(max_length=50) age = models.IntegerField() def __str__(self): return f"{self.first_name} {self.last_name}"
2. Proxy Model (Employee
): We will create a proxy model named Employee
based on the Person
model.
# app/models.py class Employee(Person): class Meta: proxy = True def get_employee_info(self): return f"{self.first_name} {self.last_name} - Age: {self.age}"
In this example:
- Meta class with
proxy = True
: This indicates thatEmployee
is a proxy model based onPerson
. - Additional method (
get_employee_info
): We’ve added a new methodget_employee_info
to theEmployee
proxy model. Proxy models can have their own methods and properties in addition to those inherited from the original model.
3. Using the Proxy Model: Now, we can use the Employee
proxy model in our Django views or anywhere else in our application:
# views.py or wherever you use models from app.models import Person, Employee # Original model usage people = Person.objects.all() for person in people: print(person) # Proxy model usage employees = Employee.objects.all() # Proxy Model for emp in employees: print(emp.get_employee_info()) # Method defined in Proxy Model
Summary
- Proxy models in Django allow you to create a new Python class that behaves almost exactly like an existing model but can have additional methods, properties, or different meta options.
- They are particularly useful when you want to add specific methods or customize behavior without altering the original model.
If you’ve enjoyed this blog post and found it insightful, there’s more where that came from! Follow us to dive deeper into various topics
Source: Django Documentation