Python strings are a fundamental data type used to represent textual data. They offer a rich set of methods and operations for string manipulation, making them indispensable in various programming tasks. In this comprehensive guide, we will explore both basic and advanced operations on Python strings to help you become proficient in string handling.
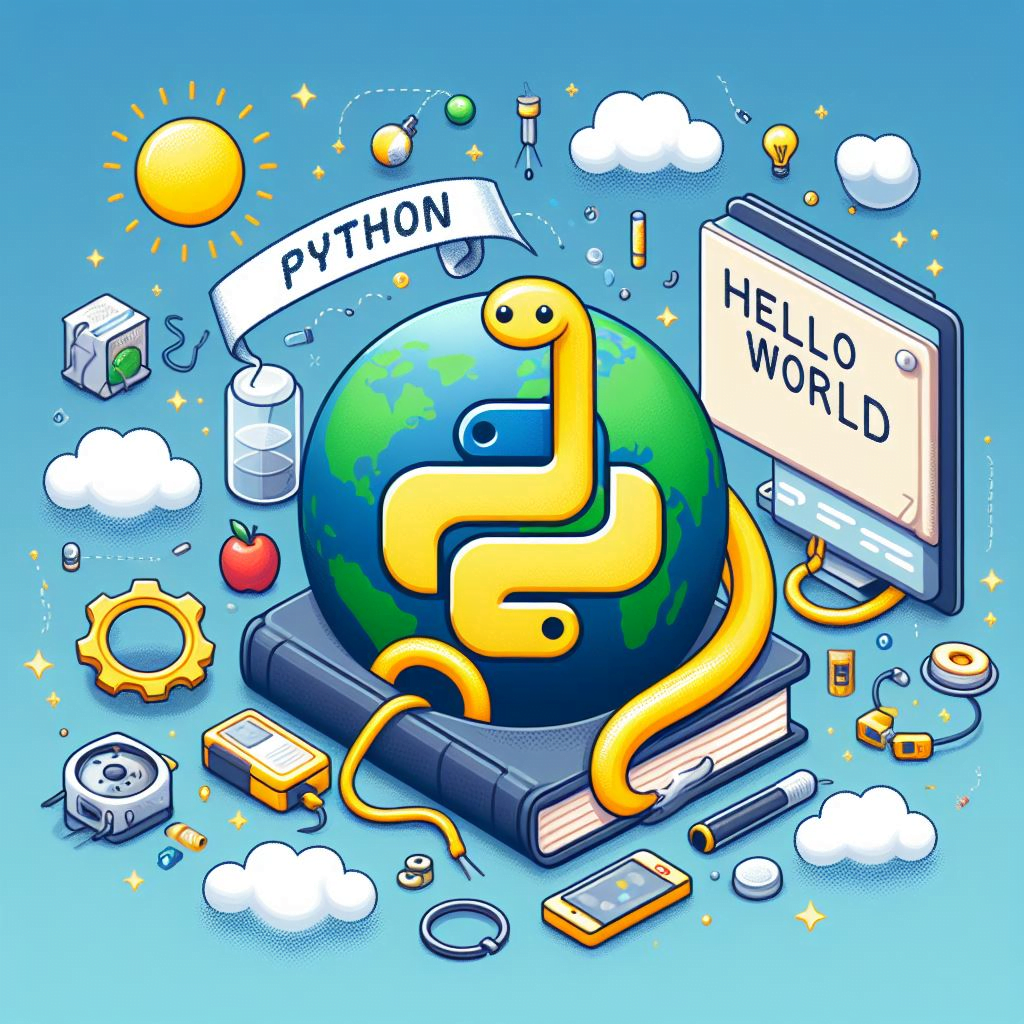
Table of Contents
- What are Python Strings?
- Basic Python Strings Operations
- Advanced String Operations
- String Formatting with f-strings
- String Methods
- String Alignment
- String Partitioning
- String Formatting with str.format()
- Escape Sequences
- String Encoding and Decoding
- String count() Method
- String maketrans() and translate() Methods
- String swapcase() Method
- String zfill() Method
- String isnumeric(), isalpha(), and isalnum() Methods
- String expandtabs() Method
- String rpartition() Method
- String title() Method
- String startswith() and endswith() Methods
- String rjust() Method with Padding
- Conclusion
What are Python Strings?
A string in Python is a sequence of characters enclosed within single quotes, double quotes, or triple quotes. Strings in Python are immutable, implying that once they are created, their content cannot be changed.
# Example of a Python string message = "Hello, World!"
Basic Python Strings Operations
String Creation
Creating a string in Python is straightforward. You can use single quotes, double quotes, or triple quotes for multi-line strings.
# Single line string single_line = "Hello, World!" # Multi-line string multi_line = '''Hello, World!'''
Accessing Characters
You can retrieve individual characters from a string using indexing.
# Accessing characters print(message[0]) # Output: H print(message[7]) # Output: W
String Slicing
String slicing allows you to extract a substring from a string.
# String slicing print(message[0:5]) # Output: Hello
String Concatenation
You can concatenate two or more strings using the + operator.
# String concatenation greeting = "Hello" name = "Alice" message = greeting + ", " + name + "!" print(message) # Output: Hello, Alice!
String Repetition
The * operator can be used to repeat a string.
# String repetition print(greeting * 3) # Output: HelloHelloHello
String Length
You can get the length of a string using the len() function.
# String length print(len(message)) # Output: 13
String Membership
You can check if a substring exists within a string using the ‘in’ and ‘not in’ operators.
# String membership print("Hello" in message) # Output: True print("world" not in message)# Output: True
Advanced String Operations
String Formatting with f-strings
F-strings offer a simple and clear way to put variables or expressions inside strings.
# Using f-strings name = "Alice" course = "BTech" print(f"Myself {name} and I am currently studying {course}.")
String Methods
Python provides a plethora of built-in methods for string manipulation.
# Common string methods print(message.upper()) # Output: HELLO, ALICE! print(message.lower()) # Output: hello, alice! print(message.strip()) # Output: Hello, Alice!
String Alignment
Python provides methods to align strings to the left, right, or center within a specified width.
# String alignment print(message.ljust(20)) # Left alignment print(message.rjust(20)) # Right alignment print(message.center(20)) # Center alignment
String Partitioning
The partition() method divides a string at the first appearance of a given separator.
# String partitioning print(message.partition(', ')) # Output: ('Hello', ', ', 'Alice!')
String Formatting with str.format()
The str.format() method offers a flexible way to format strings using placeholders.
# Using str.format() name = "Alice" course = "BTech" print("Myself {} and I am currently studying {}.".format(name, course))
Escape Sequences
Escape sequences let you add special characters to strings.
# Escape sequences print("Hello\nWorld!") # Output: # Hello # World!
String Encoding and Decoding
Python provides methods to encode and decode strings to and from different encodings.
# String encoding and decoding text = "Hello, World!" encoded_text = text.encode('utf-8') print("Encoded: ", encoded_text) # Output: b'Hello, World!' decoded_text = encoded_text.decode('utf-8') print(decoded_text) # Output: Hello, World!
String count() Method
The count() method tells you how many times a specific substring appears in a string.
# Using count() method print(message.count('Alice')) # Output: 1
String maketrans() and translate() Methods
These methods are used for mapping and translating characters in a string.
# Using maketrans() and translate() methods table = str.maketrans('aeiou', '12345') translated_message = message.translate(table) print(translated_message) # Output: H2ll4, W4rld!
String swapcase() Method
The swapcase() method gives you a new string where uppercase letters become lowercase and vice versa.
# Using swapcase() method print(message.swapcase()) # Output: hELLO, wORLD!
String zfill() Method
The zfill() method pads a numeric string with zeros on the left to fill a specified width.
# Using zfill() method number = "42" print(number.zfill(5)) # Output: 00042
String isnumeric(), isalpha(), and isalnum() Methods
These methods check if all the characters in the string are numeric, alphabetic, or alphanumeric, respectively.
# Using isnumeric(), isalpha(), and isalnum() methods numeric_string = "12345" alpha_string = "abcde" alnum_string = "abc123" print(numeric_string.isnumeric()) # Output: True print(alpha_string.isalpha()) # Output: True print(alnum_string.isalnum()) # Output: True
String expandtabs() Method
The expandtabs() method replaces tab characters (‘\t’) with spaces, using a specified tab size.
# Using expandtabs() method message = "Hello\tWorld!" print(message.expandtabs(4)) # Output: Hello World!
String rpartition() Method
The rpartition() method works similar to partition(), but it searches from the right and splits at the last occurrence of the specified separator.
# Using rpartition() method message = "Hello, World!" print(message.rpartition(', ')) # Output: ('Hello', ', ', 'World!')
String title() Method
The title() method returns a copy of the string where the first character of each word is capitalized.
# Using title() method message = "hello world" print(message.title()) # Output: Hello World
String startswith() and endswith() Methods
These methods determine if a string begins or finishes with a particular substring.
# Using startswith() and endswith() methods message = "Hello, World!" print(message.startswith("Hello")) # Output: True print(message.endswith("World!")) # Output: True
String rjust() Method with Padding
The rjust() method can also take an optional padding character as an argument.
# Using rjust() method with padding character message = "Python" print(message.rjust(10, '*')) # Output: ****Python
Conclusion
Python strings offer a versatile and powerful set of tools for string manipulation, catering to both basic and advanced requirements. From simple string creation and manipulation to more complex operations like formatting, encoding, and translation, Python provides a comprehensive suite of methods and functions to handle strings efficiently.
Understanding these operations will equip you with the necessary skills to tackle various string-related tasks effectively in Python. With this comprehensive guide, you’re well-equipped to leverage the full capabilities of strings in your Python projects. Happy coding!