Django models form the backbone of any Django application, defining the structure of your data and enabling powerful interactions with your database. Beyond basic CRUD operations (Create, Read, Update, Delete), Django models offer hooks—methods like clean()
, save()
, and delete()
—that allow developers to customize and control data validation, manipulation, and persistence at various stages of the model’s lifecycle.
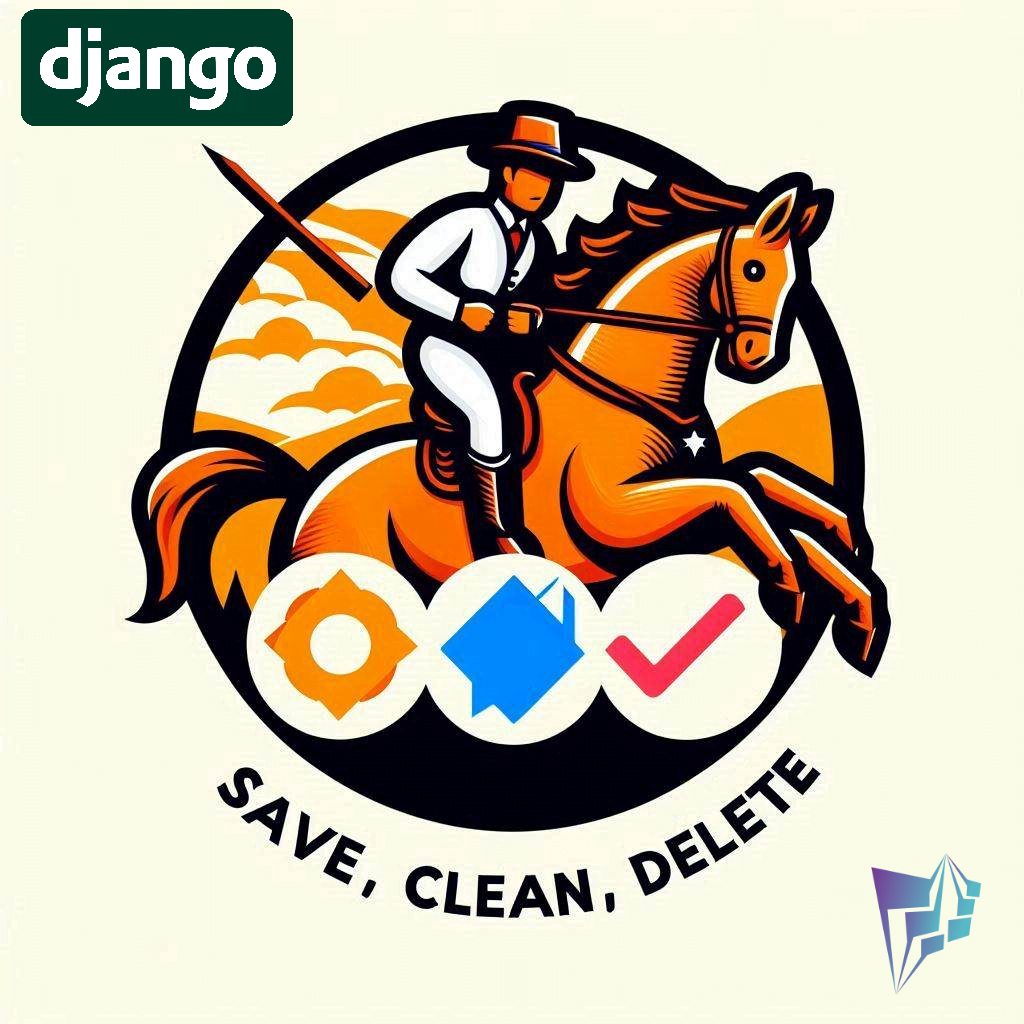
Table of Contents
- 1. clean(): Data Validation and Integrity Checks
- 2. save(): Customizing Save Behavior
- 3. delete(): Customizing Deletion Behavior
- Conclusion
1. clean()
: Data Validation and Integrity Checks
The clean()
method in Django models provides a powerful way to validate and clean data before it gets saved. This method is called during the validation process triggered by forms or model instances. By overriding clean()
, you can ensure that your data meets specific business rules or integrity constraints.
from django.db import models from datetime import datetime from django.core.validators import ValidationError class Musician(models.Model): first_name = models.CharField(max_length=100, null=True, blank=True) last_name = models.CharField(max_length=100, null=True, blank=True) instrument = models.CharField(max_length=100, null=True, blank=True) class Meta: ordering = ('first_name',) db_table = 'musician' db_table_comment = "Musician Table" def clean(self): # self contains Musician Object if self.first_name.lower() == 'test': raise ValidationError("Last Name cannot be test") if last_name.lower() == 'test': raise ValidationError("Last Name cannot be test") super(Musician, self).clean()
2. save()
: Customizing Save Behavior
The save()
method is where you can customize how your model instance is saved to the database. This is useful for preprocessing data, handling relationships, or enforcing additional business logic before persistence.
from django.db import models from datetime import datetime import logging logger = logging.getLogger(__name__) class Musician(models.Model): first_name = models.CharField(max_length=100, null=True, blank=True) last_name = models.CharField(max_length=100, null=True, blank=True) instrument = models.CharField(max_length=100, null=True, blank=True) def save(self, *args, **kwargs): # self contains musician object print(self.first_name) self.first_name = self.first_name.upper() # Convert First Name to Uppercase self.last_name = self.last_name.upper() # Convert Last Name to Uppercase logger.info(f"Saving instance with name {self.first_name} {self.last_name}") super(Musician, self).save(*args, **kwargs)
3. delete()
: Customizing Deletion Behavior
The delete()
method allows you to define custom actions when an instance of your model is deleted. This could involve cleaning up related objects, logging the deletion, or enforcing deletion constraints.
from django.db import models from datetime import datetime import logging logger = logging.getLogger(__name__) class Musician(models.Model): first_name = models.CharField(max_length=100, null=True, blank=True) last_name = models.CharField(max_length=100, null=True, blank=True) instrument = models.CharField(max_length=100, null=True, blank=True) def delete(self, *args, **kwargs): # Before Deletion logger.info(f"Deleting instance with name {self.first_name} {self.last_name}") super(Musician, self).delete(*args, **kwargs)
Conclusion
Understanding and utilizing these methods effectively empowers Django developers to implement sophisticated data validation, manipulation, and persistence logic directly within their models. Whether you’re enforcing complex validation rules, preprocessing data before saving, or managing related objects during deletion, Django’s model methods provide the flexibility and control needed to build robust web applications.
By mastering clean()
, save()
, and delete()
, developers can ensure their Django applications not only meet functional requirements but also maintain data integrity and consistency throughout the application lifecycle.
Django Model’s API Documentation
If you’ve enjoyed this blog post and found it insightful, there’s more where that came from! Follow us to dive deeper into various topics