Exception handling is a crucial aspect of any programming language, and Python is no exception (pun intended). Exception handling allows you to gracefully manage and respond to unexpected errors or exceptional situations that may arise during the execution of a program. In this blog post, we’ll delve into the basics of exception handling in Python, explore the try, except, else, and finally blocks, and provide real-life examples to illustrate these concepts.
Table of Contents
- Understanding Exceptions in Python
- Basic Exception Handling with try and except
- Handling Multiple Exceptions
- Using else and finally Blocks
- Real-Life Examples
- Overriding Exception Class
- Conclusion
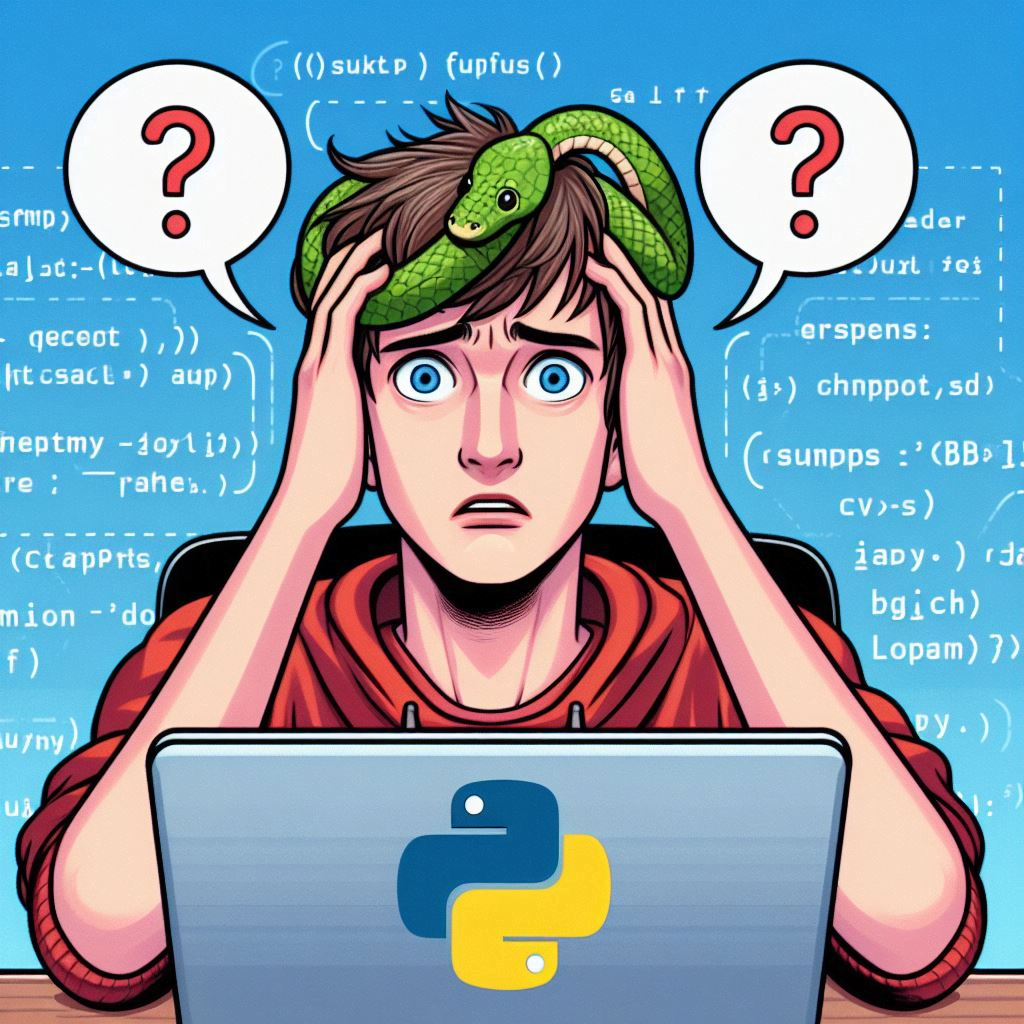
Understanding Exceptions in Python
In Python, an exception is a problem that stops a program from running normally. Common types of exceptions include ZeroDivisionError, TypeError, ValueError, and FileNotFoundError, among others. When an exception occurs, Python raises an exception, which, if not handled, will terminate the program and display an error message.
Basic Exception Handling with try and except
The try and except blocks are used together to catch and handle exceptions in Python.
try: result = 10 / 0 except ZeroDivisionError: print("Error: Cannot divide by zero")
In this example, the try block attempts to divide 10 by 0, which will raise a ZeroDivisionError. The except block catches this exception, and a custom error message is displayed instead of the default Python error message.
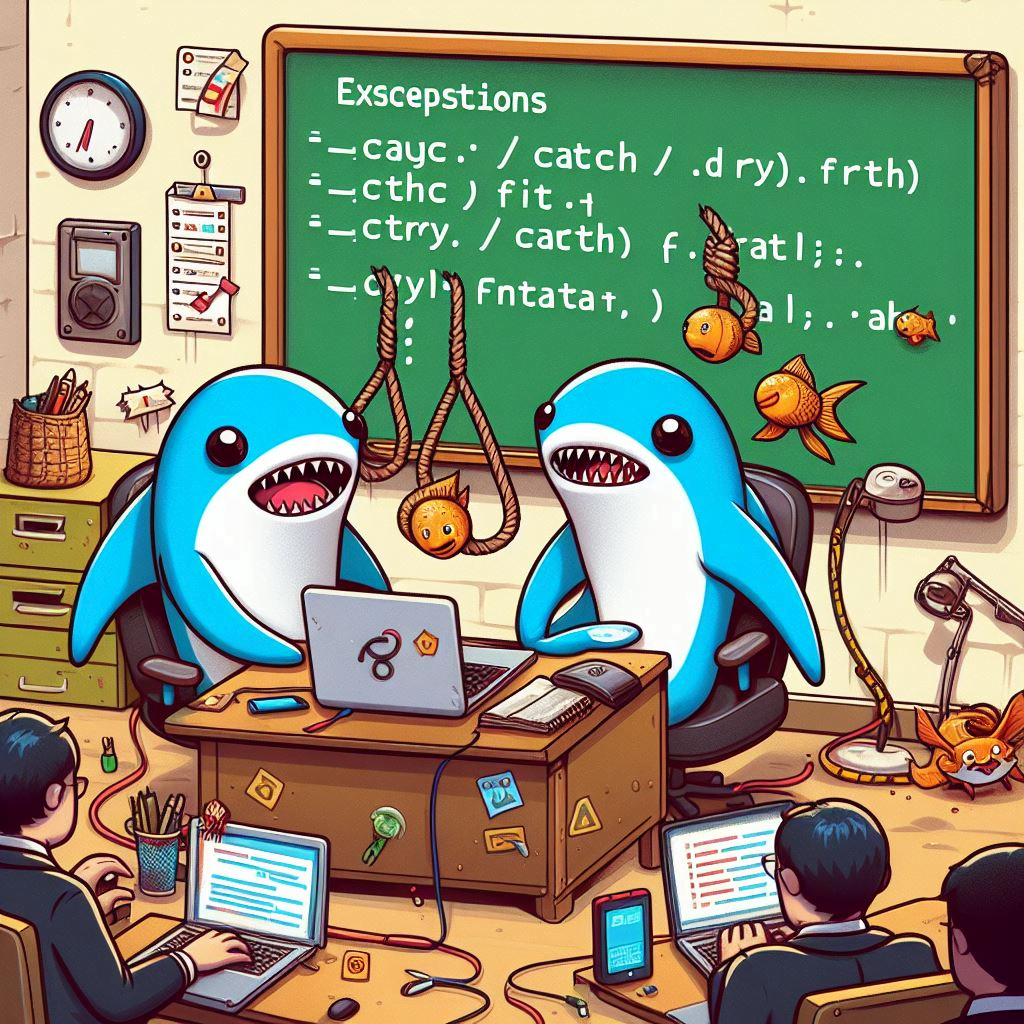
Handling Multiple Exceptions
You can handle multiple exceptions by specifying multiple except blocks or using a single except block with multiple exception types.
try: result = int("abc") except ValueError: print("Error: Invalid value") except TypeError: print("Error: Type mismatch")
Using else and finally Blocks
else Block
The else block is executed if the try block does not raise any exceptions.
try: result = 10 / 2 except ZeroDivisionError: print("Error: Cannot divide by zero") else: print(f"Result: {result}")
finally Block
The finally block runs no matter if there’s an error or not. It’s usually used to do tasks like closing files or freeing up resources.
try: file = open("example.txt", "r") # Code to read the file except FileNotFoundError: print("Error: File not found") finally: file.close()
Real-Life Examples
Example 1: Handling File Not Found
try: with open("nonexistent.txt", "r") as file: content = file.read() except FileNotFoundError: print("Error: File not found")
Example 2: Handling Invalid Input
try: age = int(input("Enter your age: ")) except ValueError: print("Error: Please enter a valid age")
Example 3: Handling Division by Zero
try: num1 = int(input("Enter the numerator: ")) num2 = int(input("Enter the denominator: ")) result = num1 / num2 except ZeroDivisionError: print("Error: Cannot divide by zero") else: print(f"Result: {result}")
Example 4: Module Import Error Handling
try: import non_existent_module except ImportError: print("Module not found!")
Overriding Exception Class
Overriding the Exception class in Python involves creating a subclass of the built-in Exception
class and customizing its behavior by overriding its methods. The most commonly overridden method is __str__
, which is responsible for generating the string representation of the exception.
Here’s how you can override the Exception
class with a custom message:
class CustomException(Exception): def __init__(self, message): super().__init__(message) self.message = message def __str__(self): return f'CustomException: {self.message}' # Example usage try: raise CustomException("This is a custom exception message.") except CustomException as e: print(e)
In this example:
- We define a custom exception class
CustomException
that inherits from the baseException
class. - In the
__init__
method, we initialize the exception with a custom message and call the__init__
method of the base class usingsuper().__init__(message)
. - We store the custom message as an instance attribute
self.message
. - We override the
__str__
method to return a string representation of the exception, including the custom message.
When an instance of CustomException
is raised and caught, the overridden __str__
method is invoked to generate the error message, providing a customized representation of the exception.
This approach allows you to create custom exception classes tailored to your specific use cases and provides flexibility in defining the behavior and error messages associated with those exceptions.
Conclusion
By understanding how to use try, except, else, and finally blocks effectively, you can write more robust and error-tolerant code. Whether you’re working on a simple script or a complex application, incorporating exception handling can help you anticipate and manage potential errors, ensuring a smoother and more reliable user experience.