In the vast landscape of Python programming, composition emerges as a fundamental concept in object-oriented design, offering developers a powerful tool to create robust, modular, and maintainable code. Through the clever combination of simpler components, composition empowers developers to build complex objects with ease, promoting code reusability, flexibility, and clarity. In this comprehensive guide, we embark on an exploration of composition in Python, unraveling its principles, applications, and benefits through a series of illustrative examples.
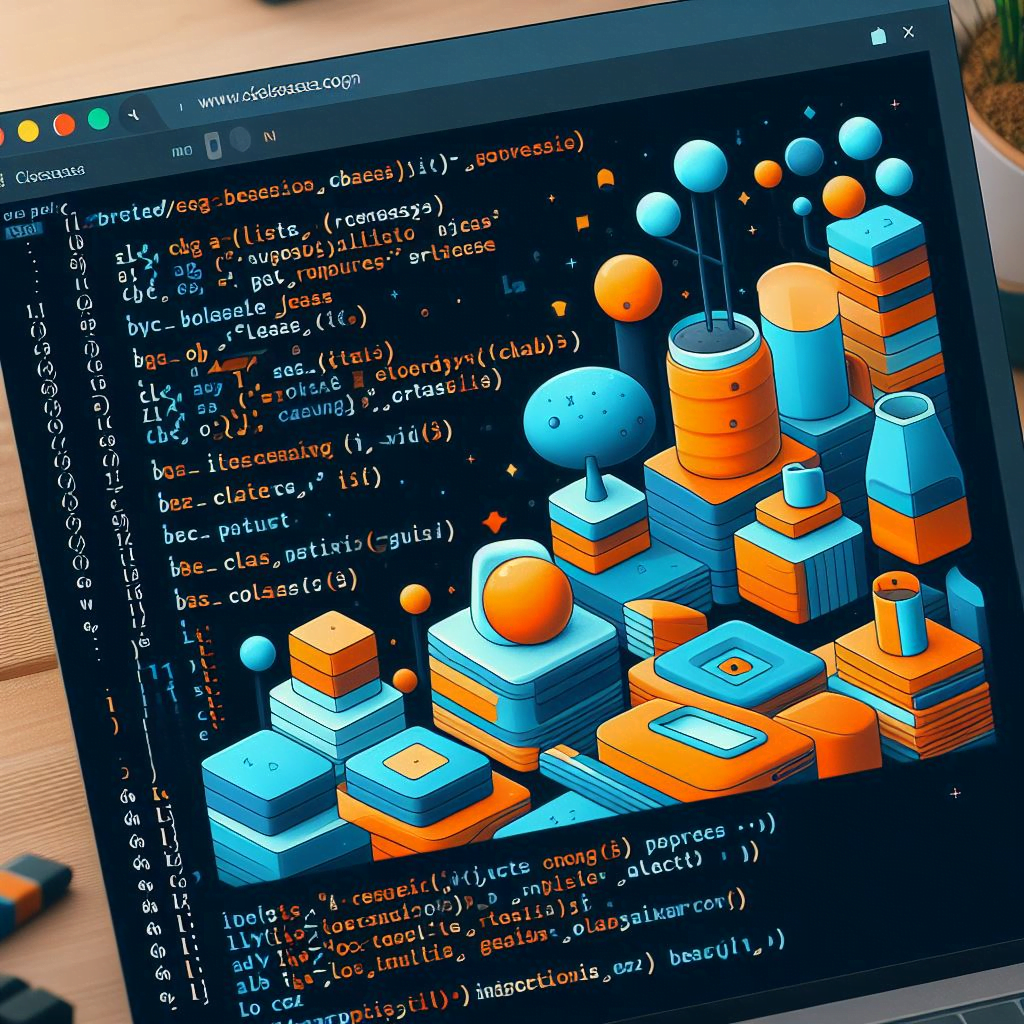
Table of Contents
- Understanding Composition: A Foundation of Object-Oriented Design
- Composing a Car: An Illustrated Example
- Exploring Composition with Additional Examples
- Benefits of Composition: Empowering Python Developers
- The Role of Constructors and Destructors in Composition
- Conclusion
Understanding Composition: A Foundation of Object-Oriented Design
At its core, composition embodies the principle of building complex objects by aggregating simpler components. Unlike inheritance, which emphasizes an “is-a” relationship between classes, composition revolves around a “has-a” relationship, allowing objects to possess and utilize the functionalities of other objects. This approach not only fosters modular design but also facilitates code reuse and enhances maintainability.
Composing a Car: An Illustrated Example
Let’s delve into a concrete example to elucidate the concept of composition in Python. Consider a scenario where we aim to model a Car
object, comprising essential components such as an Engine
and Wheel
. Each component is represented by a distinct class, encapsulating its unique functionalities.
class Engine: def start(self): return "Engine started" class Wheel: def rotate(self): return "Wheel rotating" class Car: def __init__(self): self.engine = Engine() self.wheel = Wheel() def start_car(self): return self.engine.start() def rotate_wheel(self): return self.wheel.rotate()
In this example, the Car
class utilizes composition to integrate instances of the Engine
and Wheel
classes as its constituent components. Through composition, the Car
object can seamlessly leverage the functionalities encapsulated within its internal components, such as starting the engine or rotating the wheels.
Exploring Composition with Additional Examples
Let’s further explore the versatility of composition through a variety of examples, demonstrating its applicability in different scenarios.
Example 1: Building a House
Suppose we want to model a House
object, consisting of various rooms such as a living room, bedroom, and kitchen. Each room is represented by a separate class.
class LivingRoom: def __init__(self): self.furniture = ['sofa', 'coffee table', 'TV'] class Bedroom: def __init__(self): self.furniture = ['bed', 'dresser', 'nightstand'] class Kitchen: def __init__(self): self.appliances = ['refrigerator', 'stove', 'microwave'] class House: def __init__(self): self.living_room = LivingRoom() self.bedroom = Bedroom() self.kitchen = Kitchen()
In this example, the House
object is composed of instances of the LivingRoom
, Bedroom
, and Kitchen
classes, each representing a distinct part of the house. Through composition, the House
object encapsulates the functionalities and attributes of its constituent rooms, providing a cohesive representation of a complete dwelling.
Example 2: Creating a Computer
Let’s imagine we want to model a Computer
object, comprising essential components such as a CPU, memory, and storage. Each component is represented by a separate class.
class CPU: def execute(self): return "CPU executing instructions" class Memory: def read(self): return "Memory reading data" class Storage: def write(self): return "Storage writing data" class Computer: def __init__(self): self.cpu = CPU() self.memory = Memory() self.storage = Storage() def process_data(self): instruction = self.memory.read() return self.cpu.execute(instruction) def store_data(self, data): return self.storage.write(data)
In this example, the Computer
object employs composition to incorporate instances of the CPU
, Memory
, and Storage
classes as its internal components. Through composition, the Computer
object can seamlessly execute instructions, read data from memory, and write data to storage, leveraging the functionalities of its constituent components.
Benefits of Composition: Empowering Python Developers
Composition offers a myriad of benefits, empowering Python developers to write cleaner, more modular, and more maintainable code:
- Flexibility: By assembling objects from smaller components, composition enables developers to easily modify and extend the behavior of complex objects.
- Code Reusability: Leveraging composition promotes code reuse, as smaller components can be shared across multiple objects and projects.
- Modularity: Composition encourages modular design, allowing developers to break down complex systems into smaller, manageable parts.
- Clarity and Maintainability: Through composition, code becomes more understandable and maintainable, as each component encapsulates a specific functionality or feature.
The Role of Constructors and Destructors in Composition
In Python, constructors (__init__
method) and destructors (__del__
method) play a crucial role in initializing and cleaning up object instances. Let’s explore their significance in the context of composition.
class MyClass: def __init__(self): print("Constructor called") def __del__(self): print("Destructor called") # Creating objects obj1 = MyClass() # Output: Constructor called obj2 = MyClass() # Output: Constructor called # Deleting objects del obj1 # Output: Destructor called del obj2 # Output: Destructor called
In this example, the __init__
method serves as the constructor, responsible for initializing object instances, while the __del__
method acts as the destructor, performing cleanup operations before an object is removed from memory. In the context of composition, constructors are particularly useful for initializing the internal components of composite objects, ensuring they are properly configured and ready for use.
Conclusion
Composition stands as a cornerstone of object-oriented design in Python, offering developers a powerful mechanism to create sophisticated objects from simpler components. By embracing composition, Python developers can unlock new possibilities in software design, enabling the creation of flexible, reusable, and maintainable codebases. So, dive into composition, explore its nuances, and elevate your Python programming skills to new heights.