Python, known for its simplicity and versatility, owes much of its power to its modular architecture. Modules in Python are essentially files containing Python code, which can be imported and used in other Python scripts. In this article, we will delve into how to create and use your own modules and explore some of the most commonly used built-in modules in Python.
Table of Contents
- Creating Your Own Modules in Python
- Exploring Built-in Modules
- re module
- Conclusion
Creating Your Own Modules in Python
Creating a Python module is a straightforward process. You simply write your Python code in a .py file, and that file becomes a module. Here’s a step-by-step guide to creating your own Python module:
Write your Python code: Create a new .py file and write the Python code you want to include in your module. For example, let’s create a file named math_operations.py with the following code
# math_operations.py def add(a, b): return a + b def subtract(a, b): return a - b def multiply(a, b): return a * b def divide(a, b): if b == 0: return "Division by zero is not allowed" return a / b
Save the file: Save the .py file with an appropriate name, such as math_operations.py.
Import the module: Now, you can import the module in another Python script and use the functions defined in it. Here’s how you can do it:
# main.py import math_operations print(math_operations.add(5, 3)) # Output: 8 print(math_operations.subtract(5, 3)) # Output: 2
Exploring Built-in Modules
Python comes with a rich set of built-in modules that provide a wide range of functionalities, from mathematical operations to file I/O and more. Here’s a list of some commonly used built-in modules and their uses:
math module
The math module in Python offers a collection of functions designed for executing various mathematical computations. These functions cover a wide range of mathematical operations, including basic arithmetic, trigonometry, logarithms, and more. Below is an overview of some commonly used functions available in the math module.
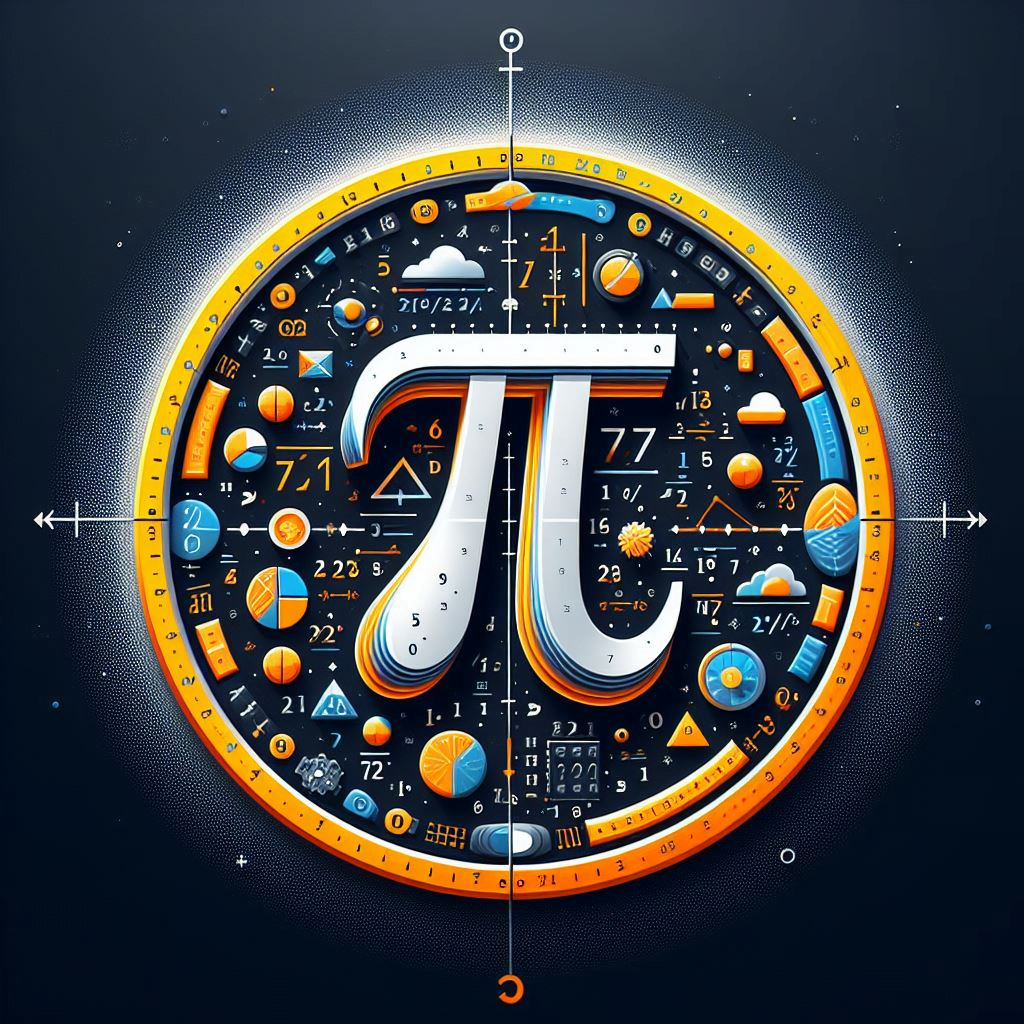
Basic Arithmetic Functions
math.ceil(x)
Returns the smallest integer value greater than or equal to x.
import math print(math.ceil(4.3)) # Output: 5 print(math.ceil(-4.3)) # Output: -4
math.floor(x)
Returns the largest integer value less than or equal to x.
import math print(math.floor(4.7)) # Output: 4 print(math.floor(-4.7)) # Output: -5
math.trunc(x)
Returns the truncated integer value of x (removes the decimal part).
import math print(math.trunc(4.9)) # Output: 4 print(math.trunc(-4.9)) # Output: -4
Trigonometric Functions
math.sin(x)
Returns the sine of x (in radians).
import math print(math.sin(math.pi/2)) # Output: 1.0
math.cos(x)
Returns the cosine of x (in radians).
import math print(math.cos(0)) # Output: 1.0
math.tan(x)
Returns the tangent of x (in radians).
import math print(math.tan(math.pi/4)) # Output: 0.9999999999999999
Exponential and Logarithmic Functions
math.exp(x)
Returns the exponential of x (e^x).
import math print(math.exp(1)) # Output: 2.718281828459045
math.log(x[, base])
Returns the natural logarithm of x (base e). Optionally, you can specify the base for the logarithm.
import math print(math.log(2)) # Output: 0.6931471805599453 print(math.log(8, 2)) # Output: 3.0
math.sqrt(x)
Returns the square root of x.
import math print(math.sqrt(16)) # Output: 4.0
Constants
math.pi
A mathematical constant representing π (pi).
import math print(math.pi) # Output: 3.141592653589793
math.e
A mathematical constant representing the base of natural logarithms, approximately equal to 2.71828.
import math print(math.e) # Output: 2.718281828459045
math.inf
A floating-point representation of positive infinity.
import math print(math.inf) # Output: inf
math.nan
A floating-point representation of NaN (Not a Number).
import math print(math.nan) # Output: nan
These are just a few examples of the functions available in the math module.
datetime module
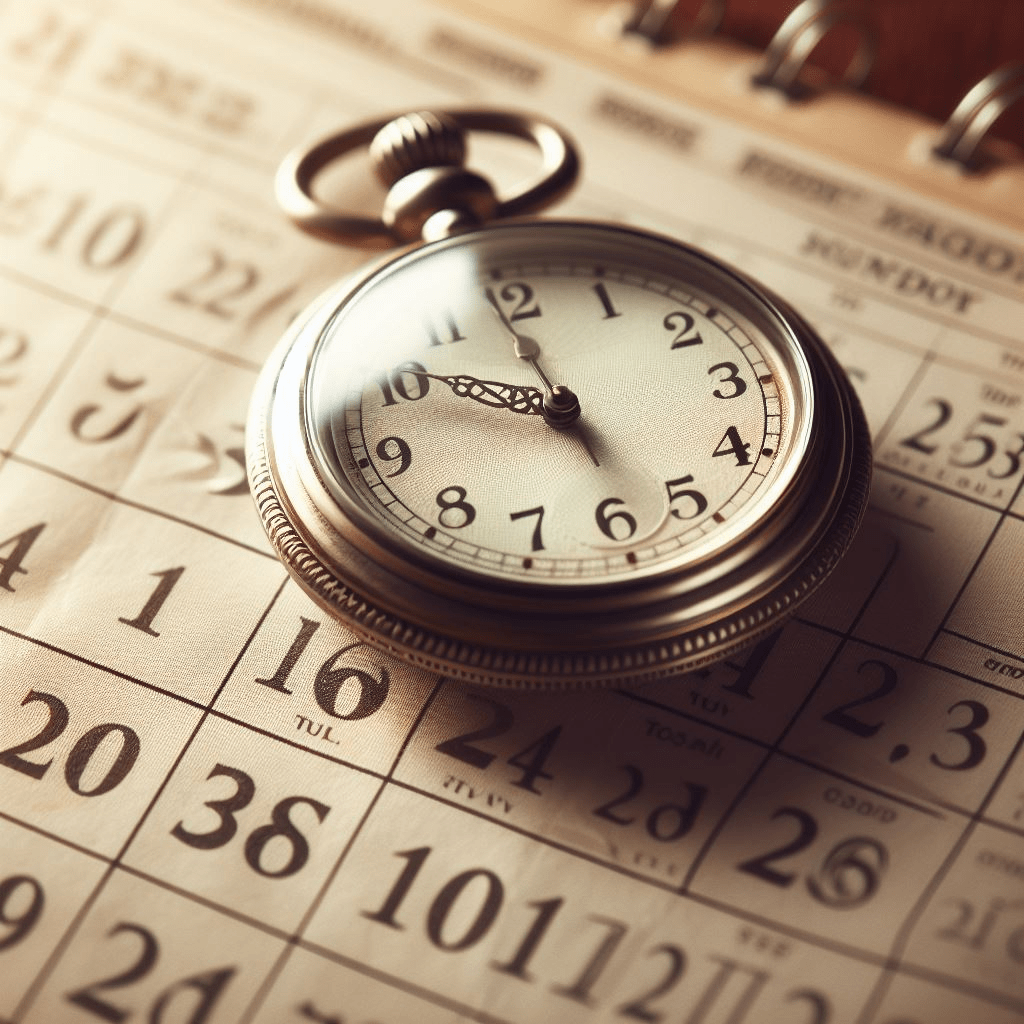
The datetime module in Python provides functionalities for managing dates and times. It offers various functionalities for creating, formatting, and performing arithmetic operations on dates and times. Below is an in-depth look at the datetime module, focusing on the datetime class and its methods.
datetime class
The datetime class in the datetime module represents a specific point in time with both date and time components. It is a combination of a date object and a time object.
Creating datetime objects
You can create a datetime object using the datetime class constructor, which takes year, month, day, hour, minute, second, microsecond, and timezone information as arguments.
from datetime import datetime # Create a datetime object for a specific date and time dt = datetime(2024, 4, 21, 12, 30, 45) print(dt) # Output: 2024-04-21 12:30:45
Getting Current Date and Time
The datetime.now() method returns the current local date and time.
from datetime import datetime current_dt = datetime.now() print(current_dt) # Output: Current local date and time
Getting Date and Time Components
You can access individual components of a datetime object, such as year, month, day, hour, minute, second, and microsecond.
from datetime import datetime dt = datetime.now() print(f"Year: {dt.year}") print(f"Month: {dt.month}") print(f"Day: {dt.day}") print(f"Hour: {dt.hour}") print(f"Minute: {dt.minute}") print(f"Second: {dt.second}") print(f"Microsecond: {dt.microsecond}")
datetime class methods
date()
The date() method returns a date object with the same year, month, and day components as the datetime object.
from datetime import datetime dt = datetime.now() date_only = dt.date() print(date_only) # Output: YYYY-MM-DD
time()
The time() method returns a time object with the same hour, minute, second, and microsecond components as the datetime object.
from datetime import datetime dt = datetime.now() time_only = dt.time() print(time_only) # Output: HH:MM:SS.microsecond
strftime()
The strftime() method formats the datetime object as a string according to a specified format.
from datetime import datetime dt = datetime.now() formatted_dt = dt.strftime("%Y-%m-%d %H:%M:%S") print(formatted_dt) # Output: YYYY-MM-DD HH:MM:SS
strptime()
The strptime() method creates a datetime object from a string representing a date and time, given a corresponding format string.
from datetime import datetime date_str = "2024-04-21 12:30:45" dt = datetime.strptime(date_str, "%Y-%m-%d %H:%M:%S") print(dt) # Output: 2024-04-21 12:30:45
Arithmetic Operations
You can perform arithmetic operations on datetime objects to calculate time differences or add/subtract time intervals.
from datetime import datetime, timedelta dt1 = datetime(2024, 4, 21) dt2 = datetime(2024, 4, 25) # Calculate the difference between two dates delta = dt2 - dt1 print(delta.days) # Output: 4 # Add a time interval to a datetime object new_dt = dt1 + timedelta(days=10) print(new_dt) # Output: 2024-05-01 00:00:00
os module
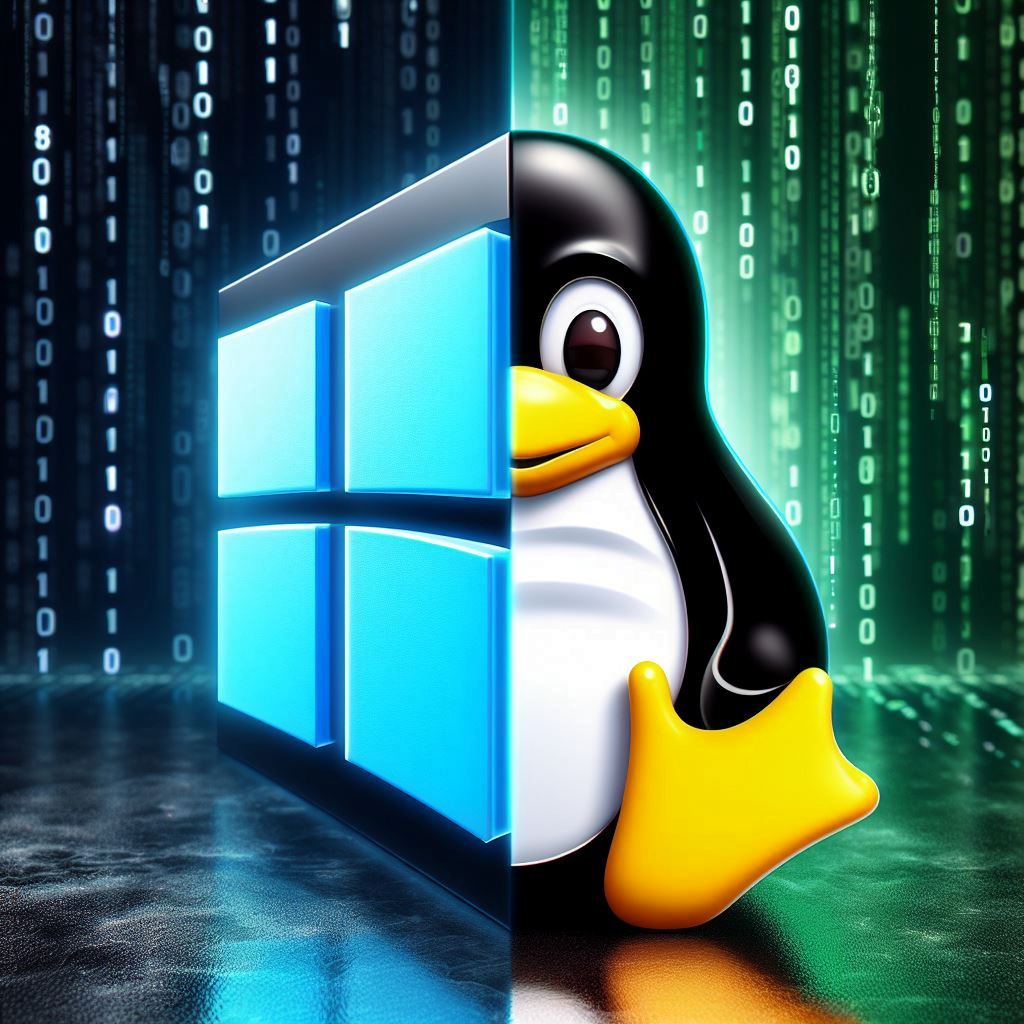
The os module in Python offers tools for interacting with the operating system. It offers a range of functions for performing file and directory operations, accessing environment variables, and executing system commands. Below are some of the key functionalities provided by the os module:
File and Directory Operations
Checking if a File or Folder Exists
You can use the os.path.exists() function to check if a file or directory exists at a given path.
import os file_path = 'example.txt' if os.path.exists(file_path): print(f"{file_path} exists.") else: print(f"{file_path} does not exist.")
Creating and Removing Directories
The os.mkdir() and os.rmdir() functions are used to create and remove directories, respectively.
import os # Create a directory os.mkdir('my_directory') # Remove a directory os.rmdir('my_directory')
Listing Files and Directories
You can use the os.listdir() function to get a list of all files and directories in a given directory.
import os files = os.listdir('.') for file in files: print(file)
Operating System Information
Getting Current Working Directory
The os.getcwd() function provides the current working directory as a string.
import os cwd = os.getcwd() print(f"Current working directory: {cwd}")
Changing Current Working Directory
The os.chdir() function allows you to change the current working directory.
import os os.chdir('/path/to/directory') print(f"Current working directory changed to {os.getcwd()}")
Platform Information
The os.name attribute provides the name of the operating system dependent module imported. The os.uname() function returns information identifying the current operating system.
import os print(f"OS name: {os.name}") if os.name == 'posix': print(f"Platform: {os.uname()}")
Environment Variables
Accessing Environment Variables
The os.environ dictionary provides access to the environment variables of the system.
import os # Accessing the PATH environment variable path = os.environ.get('PATH') print(f"PATH: {path}")
System Commands
Running System Commands
The os.system() function allows you to execute shell commands.
import os # Run the 'ls' command (Unix/Linux) os.system('ls') # Run the 'dir' command (Windows) os.system('dir')
File and Path Operations
Path Manipulation
The os.path module provides functions for manipulating paths and checking path-related information.
import os # Joining paths path = os.path.join('dir1', 'dir2', 'file.txt') print(f"Joined path: {path}") # Getting the basename and dirname print(f"Basename: {os.path.basename(path)}") print(f"Dirname: {os.path.dirname(path)}") # Splitting the extension print(f"Splitext: {os.path.splitext(path)}")
sys module
The sys module provides access to some variables used or maintained by the interpreter and to functions that interact with the interpreter.
import sys print(sys.platform) # Output: linux
random module
The main function of the random module is to produce random numbers, sequences, and selections. Whether you need to simulate random events, shuffle data, or generate cryptographic keys, the random module offers a wide range of functionalities to meet your needs.
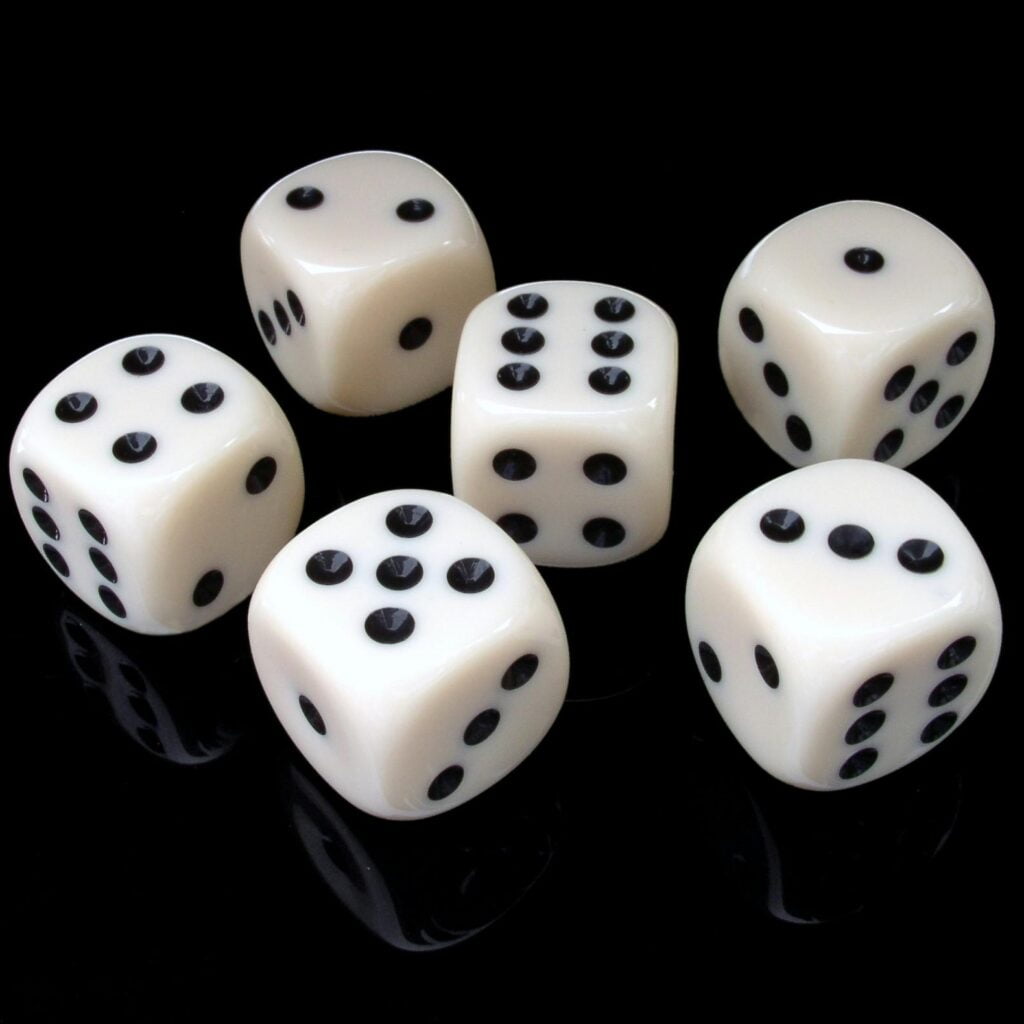
Generating Random Numbers
One of the primary purposes of the random module is to generate random numbers. The random.randint() function is commonly used to generate a random integer within a specified range, inclusive of both endpoints.
import random random_number = random.randint(1, 10) print(random_number) # Output: a random integer between 1 and 10
Generating Random Floats
In addition to integers, the random module also allows you to generate random floating-point numbers using the random.uniform() function.
import random random_float = random.uniform(1.0, 10.0) print(random_float) # Output: a random float between 1.0 and 10.0
Generating Random Sequences
The random module provides functions for generating random sequences, such as lists, using the random.sample() function.
import random random_list = random.sample(range(1, 10), 3) print(random_list) # Output: a list of 3 unique random numbers between 1 and 10
Shuffling Sequences
The random module also offers the random.shuffle() function, which allows you to shuffle the elements of a sequence in place.
import random my_list = [1, 2, 3, 4, 5] random.shuffle(my_list) print(my_list) # Output: a shuffled version of my_list
Choosing Random Elements
If you need to select random elements from a sequence without replacement, you can use the random.choice() function.
import random my_list = ['apple', 'banana', 'cherry', 'mango'] random_fruit = random.choice(my_list) print(random_fruit) # Output: a random fruit from my_list
Seed for Reproducibility
For applications where reproducibility is essential, the random module allows you to set a seed using the random.seed() function. Setting a seed ensures that the sequence of random numbers generated is the same every time the code is executed.
import random random.seed(42) print(random.randint(1, 10)) random.seed(42) print(random.randint(1, 10))
json module
The json module in Python offers a robust set of functions designed to handle the encoding and decoding of JSON (JavaScript Object Notation) data. JSON is a lightweight data interchange format that is widely used for transmitting data between a server and a web application, as well as for storing configuration data and structured data.
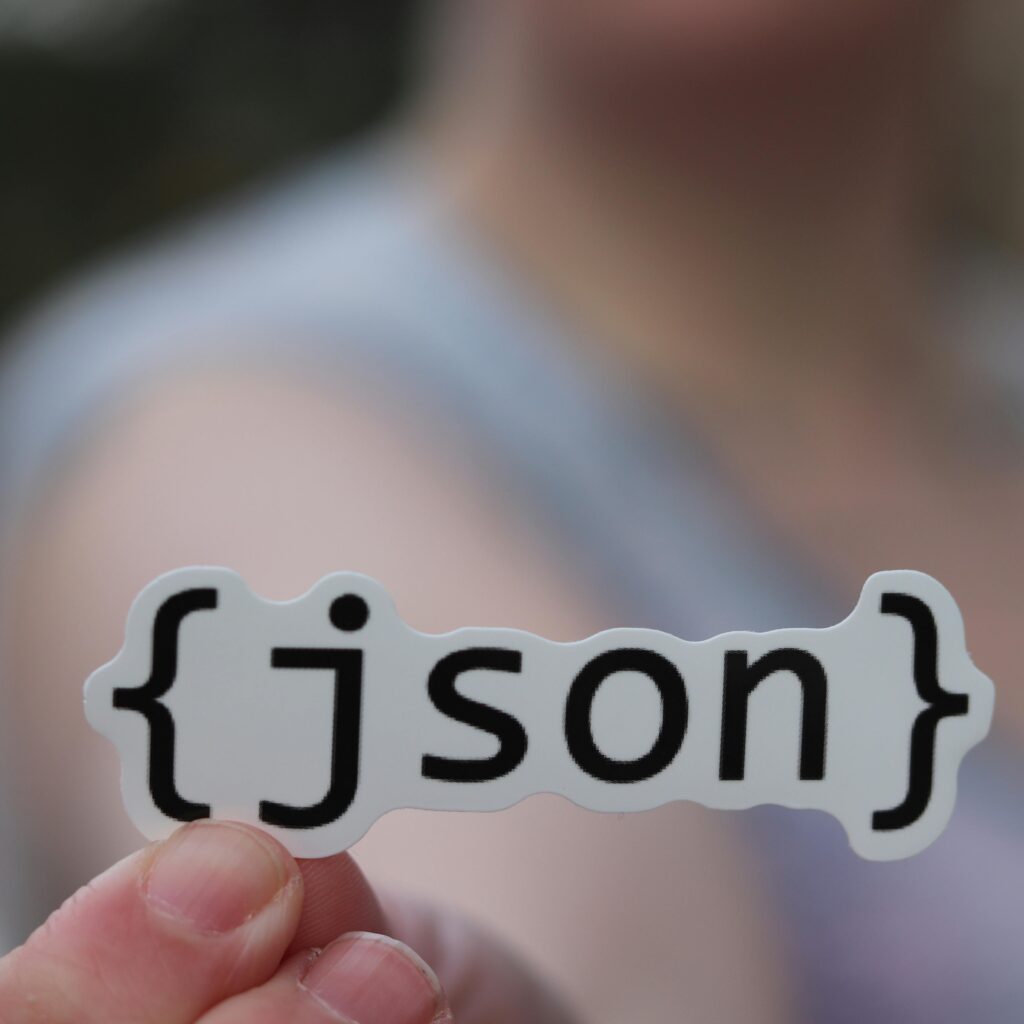
Encoding JSON Data
When it comes to encoding JSON data, the json module provides the json.dumps() function, which takes a Python object as input and returns a JSON-formatted string representation of that object. This function is particularly useful when you need to serialize Python objects into a format that can be easily transmitted or stored.
import json data = {"name": "John", "age": 30} json_data = json.dumps(data) print(json_data) # Output: {"name": "John", "age": 30}
Handling JSON Files
The json module also provides functions for reading and writing JSON data directly from and to files. The json.dump() function can be used to write JSON data to a file, while the json.load() function can be used to read JSON data from a file.
import json # Writing JSON data to a file data = {"name": "John", "age": 30} with open('data.json', 'w') as f: json.dump(data, f) # Reading JSON data from a file with open('data.json', 'r') as f: loaded_data = json.load(f) print(loaded_data) # Output: {'name': 'John', 'age': 30}
Decoding JSON Data
json.loads()
is a Python function used to decode JSON (JavaScript Object Notation) formatted strings into Python objects.
import json # JSON string representing a dictionary json_string = '{"name": "John", "age": 30, "city": "New York"}' # Using json.loads() to decode the JSON string into a Python dictionary python_dict = json.loads(json_string) # Printing the Python dictionary print(python_dict)
re module
The re module in Python provides a comprehensive set of tools and functionalities specifically designed for working with regular expressions. Regular expressions, often abbreviated as regex or regexp, are powerful patterns used to match, search, and manipulate text based on certain rules or patterns. With the re module, you can perform tasks such as searching for specific patterns within strings, replacing text based on patterns, and validating strings against specific criteria defined by regular expressions. This module enables you to harness the full power of regular expressions, making text processing tasks more efficient and flexible in Python.
import re pattern = r'\b[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}\b' text = "Contact us at email@example.com" match = re.search(pattern, text, re.IGNORECASE) print(match.group()) # Output: email@example.com
To validate Aadhar Number Pattern:
import re aadhar_number = "1122 3344 5566" # ^ asserts the start of the string. # \d{4} matches exactly 4 digits. # \s? matches an optional space character. # $ asserts the end of the string. if re.match(r"^\d{4}\s?\d{4}\s?\d{4}$", aadhar_number): print("Valid Aadhar Number") else: print("Invalid Aadhar Number")
To validate PAN Number Pattern:
import re # ^ asserts the start of the string. # [A-Z]{5} matches exactly 5 uppercase alphabetic characters. # [0-9]{4} matches exactly 4 digits. # [A-Z] matches exactly 1 uppercase alphabetic character. # $ asserts the end of the string. pan_number = "ABCDE1234F" if re.match(r"^[A-Z]{5}[0-9]{4}[A-Z]$", pan_number): print("Valid PAN Number") else: print("Invalid PAN Number")
Conclusion
Modules in Python offer a powerful way to organize and reuse code. Whether you are creating your own modules or leveraging the built-in modules provided by Python, understanding how to work with modules can greatly enhance your Python programming experience. So, go ahead, create your own modules, and explore the vast array of built-in modules to unlock the full potential of Python.